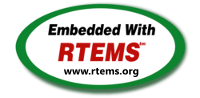 |
RTEMS
5.1
|
Go to the documentation of this file. 19 #ifndef _RTEMS_ZILOG_Z8530_H 20 #define _RTEMS_ZILOG_Z8530_H 28 #define VOL8( ptr ) ((volatile uint8_t *)(ptr)) 30 #define Z8x30_STATE0 ( z8530 ) \ 32 (garbage) = *(VOL8(z8530)) \ 35 #define Z8x30_WRITE_CONTROL( z8530, reg, data ) \ 36 *(VOL8(z8530)) = (reg); \ 37 *(VOL8(z8530)) = (data) 39 #define Z8x30_READ_CONTROL( z8530, reg, data ) \ 40 *(VOL8(z8530)) = (reg); \ 41 (data) = *(VOL8(z8530)) 43 #define Z8x30_WRITE_DATA( z8530, data ) \ 44 *(VOL8(z8530)) = (data); 46 #define Z8x30_READ_DATA( z8530, data ) \ 47 (data) = *(VOL8(z8530)); 52 #define RR_0_TX_BUFFER_EMPTY 0x04 53 #define RR_0_RX_DATA_AVAILABLE 0x01