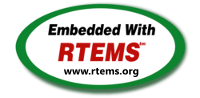 |
RTEMS
5.1
|
Go to the documentation of this file. 25 static inline void tic(
void)
30 "stw 0, ppc_tic_tac@sdarel(13);" 38 static inline uint32_t tac(
void)
44 "lwz %1, ppc_tic_tac@sdarel(13);" 46 :
"=r" (ticks),
"=r" (tmp)
54 static inline void boom(
void)
59 "stw 0, ppc_boom_bam@sdarel(13);" 67 static inline uint32_t bam(
void)
73 "lwz %1, ppc_boom_bam@sdarel(13);" 75 :
"=r" (ticks),
"=r" (tmp)