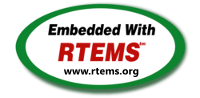 |
RTEMS
5.1
|
Go to the documentation of this file. 51 #define TFSINFOSIZE 23 54 #define TFSNAMESIZE 23 57 #ifndef TFS_CHANGELOG_FILE 58 #define TFS_CHANGELOG_SIZE 0 59 #define TFS_CHANGELOG_FILE ".tfschlog" 63 #define SYMFILE "symtbl" 70 #define TFS_RESERVED 4 75 #define TFS_EXEC 0x00000001 76 #define TFS_BRUN 0x00000002 77 #define TFS_QRYBRUN 0x00000004 79 #define TFS_SYMLINK 0x00000008 80 #define TFS_EBIN 0x00000010 81 #define TFS_CPRS 0x00000040 82 #define TFS_IPMOD 0x00000080 83 #define TFS_UNREAD 0x00000100 86 #define TFS_ULVLMSK 0x00000600 87 #define TFS_ULVL0 0x00000000 88 #define TFS_ULVL1 0x00000200 89 #define TFS_ULVL2 0x00000400 90 #define TFS_ULVL3 0x00000600 91 #define TFS_NSTALE 0x00000800 96 #define TFS_ACTIVE 0x00008000 98 #define TFS_ULVLMAX TFS_ULVL3 99 #define TFS_USRLVL(f) ((f->flags & TFS_ULVLMSK) >> 9) 102 #define TFS_RDONLY 0x00010000 103 #define TFS_CREATE 0x00020000 105 #define TFS_APPEND 0x00040000 108 #define TFS_ALLFFS 0x00080000 109 #define TFS_CREATERM 0x00100000 116 #define TFS_RCFILE "monrc" 121 #define TFS_MEMDEAD 3 127 #define TFS_MEMAVAIL 10 128 #define TFS_TIMEFUNCS 11 129 #define TFS_DOCOMMAND 12 130 #define TFS_INITDEV 13 131 #define TFS_CHECKDEV 14 132 #define TFS_DEFRAGDEV 15 133 #define TFS_DEFRAGOFF 16 134 #define TFS_DEFRAGON 17 135 #define TFS_HEADROOM 18 136 #define TFS_FCOUNT 19 143 unsigned short hdrsize;
144 unsigned short hdrvrsn;
147 unsigned long filcrc;
148 unsigned long hdrcrc;
149 unsigned long modtime;
151 char name[TFSNAMESIZE+1];
152 char info[TFSINFOSIZE+1];
154 unsigned long rsvd[TFS_RESERVED];
158 #define TFSHDRSIZ sizeof(struct tfshdr) 162 #define TFSERR_NOFILE -1 163 #define TFSERR_NOSLOT -2 164 #define TFSERR_EOF -3 165 #define TFSERR_BADARG -4 166 #define TFSERR_NOTEXEC -5 167 #define TFSERR_BADCRC -6 168 #define TFSERR_FILEEXISTS -7 169 #define TFSERR_FLASHFAILURE -8 170 #define TFSERR_WRITEMAX -9 171 #define TFSERR_RDONLY -10 172 #define TFSERR_BADFD -11 173 #define TFSERR_BADHDR -12 174 #define TFSERR_CORRUPT -13 175 #define TFSERR_MEMFAIL -14 176 #define TFSERR_NOTIPMOD -16 177 #define TFSERR_MUTEXFAILURE -17 178 #define TFSERR_FLASHFULL -18 179 #define TFSERR_USERDENIED -19 180 #define TFSERR_NAMETOOBIG -20 181 #define TFSERR_FILEINUSE -21 182 #define TFSERR_NOTCPRS -22 183 #define TFSERR_NOTAVAILABLE -23 184 #define TFSERR_BADFLAG -24 185 #define TFSERR_CLEANOFF -25 186 #define TFSERR_FLAKEYSOURCE -26 187 #define TFSERR_BADEXTENSION -27 188 #define TFSERR_MIN -100 192 #define TFS_CURRENT 2 196 #define TFS_DELETED(fp) (!((fp)->flags & TFS_ACTIVE)) 197 #define TFS_FILEEXISTS(fp) ((fp)->flags & TFS_ACTIVE) 198 #define TFS_ISCPRS(fp) ((fp)->flags & TFS_CPRS) 199 #define TFS_ISEXEC(fp) ((fp)->flags & TFS_EXEC) 200 #define TFS_ISBOOT(fp) ((fp)->flags & TFS_BRUN) 201 #define TFS_ISLINK(fp) ((fp)->flags & TFS_SYMLINK) 202 #define TFS_STALE(fp) (!((fp)->flags & TFS_NSTALE)) 203 #define TFS_FLAGS(fp) ((fp)->flags) 204 #define TFS_NAME(fp) ((fp)->name) 205 #define TFS_SIZE(fp) ((fp)->filsize) 206 #define TFS_TIME(fp) ((fp)->modtime) 207 #define TFS_INFO(fp) ((fp)->info) 208 #define TFS_NEXT(fp) ((fp)->next) 209 #define TFS_CRC(fp) ((fp)->filcrc) 210 #define TFS_ENTRY(fp) ((fp)->entry) 211 #define TFS_BASE(fp) ((char *)(fp)+(fp)->hdrsize)