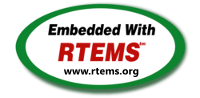 |
RTEMS
5.1
|
39 #ifndef LIBBSP_ARM_TMS570_I2C 40 #define LIBBSP_ARM_TMS570_I2C 61 uint8_t reserved1 [8];
77 #define TMS570_I2C_OAR_OA(val) BSP_FLD32(val,0, 9) 78 #define TMS570_I2C_OAR_OA_GET(reg) BSP_FLD32GET(reg,0, 9) 79 #define TMS570_I2C_OAR_OA_SET(reg,val) BSP_FLD32SET(reg, val,0, 9) 84 #define TMS570_I2C_IMR_AASEN BSP_BIT32(6) 87 #define TMS570_I2C_IMR_SCDEN BSP_BIT32(5) 90 #define TMS570_I2C_IMR_TXRDYEN BSP_BIT32(4) 93 #define TMS570_I2C_IMR_RXRDYEN BSP_BIT32(3) 96 #define TMS570_I2C_IMR_ARDYEN BSP_BIT32(2) 99 #define TMS570_I2C_IMR_NACKEN BSP_BIT32(1) 102 #define TMS570_I2C_IMR_ALEN BSP_BIT32(0) 107 #define TMS570_I2C_STR_SDIR BSP_BIT32(14) 110 #define TMS570_I2C_STR_NACKSNT BSP_BIT32(13) 113 #define TMS570_I2C_STR_BB BSP_BIT32(12) 116 #define TMS570_I2C_STR_RSFULL BSP_BIT32(11) 119 #define TMS570_I2C_STR_XSMT BSP_BIT32(10) 122 #define TMS570_I2C_STR_AAS BSP_BIT32(9) 125 #define TMS570_I2C_STR_AD0 BSP_BIT32(8) 128 #define TMS570_I2C_STR_SCD BSP_BIT32(5) 131 #define TMS570_I2C_STR_TXRDY BSP_BIT32(4) 134 #define TMS570_I2C_STR_RXRDY BSP_BIT32(3) 137 #define TMS570_I2C_STR_ARDY BSP_BIT32(2) 140 #define TMS570_I2C_STR_NACK BSP_BIT32(1) 143 #define TMS570_I2C_STR_AL BSP_BIT32(0) 148 #define TMS570_I2C_CKL_CLKL(val) BSP_FLD32(val,0, 15) 149 #define TMS570_I2C_CKL_CLKL_GET(reg) BSP_FLD32GET(reg,0, 15) 150 #define TMS570_I2C_CKL_CLKL_SET(reg,val) BSP_FLD32SET(reg, val,0, 15) 155 #define TMS570_I2C_CKH_CLKH(val) BSP_FLD32(val,0, 15) 156 #define TMS570_I2C_CKH_CLKH_GET(reg) BSP_FLD32GET(reg,0, 15) 157 #define TMS570_I2C_CKH_CLKH_SET(reg,val) BSP_FLD32SET(reg, val,0, 15) 162 #define TMS570_I2C_CNT_CNT(val) BSP_FLD32(val,0, 15) 163 #define TMS570_I2C_CNT_CNT_GET(reg) BSP_FLD32GET(reg,0, 15) 164 #define TMS570_I2C_CNT_CNT_SET(reg,val) BSP_FLD32SET(reg, val,0, 15) 169 #define TMS570_I2C_DRR_DATARX(val) BSP_FLD32(val,0, 7) 170 #define TMS570_I2C_DRR_DATARX_GET(reg) BSP_FLD32GET(reg,0, 7) 171 #define TMS570_I2C_DRR_DATARX_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 176 #define TMS570_I2C_SAR_SA(val) BSP_FLD32(val,0, 9) 177 #define TMS570_I2C_SAR_SA_GET(reg) BSP_FLD32GET(reg,0, 9) 178 #define TMS570_I2C_SAR_SA_SET(reg,val) BSP_FLD32SET(reg, val,0, 9) 183 #define TMS570_I2C_DXR_DATATX(val) BSP_FLD32(val,0, 7) 184 #define TMS570_I2C_DXR_DATATX_GET(reg) BSP_FLD32GET(reg,0, 7) 185 #define TMS570_I2C_DXR_DATATX_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 190 #define TMS570_I2C_MDR_NACKMOD BSP_BIT32(15) 193 #define TMS570_I2C_MDR_FREE BSP_BIT32(14) 196 #define TMS570_I2C_MDR_STT BSP_BIT32(13) 199 #define TMS570_I2C_MDR_STP BSP_BIT32(11) 202 #define TMS570_I2C_MDR_MST BSP_BIT32(10) 205 #define TMS570_I2C_MDR_TRX BSP_BIT32(9) 208 #define TMS570_I2C_MDR_XA BSP_BIT32(8) 211 #define TMS570_I2C_MDR_RM BSP_BIT32(7) 214 #define TMS570_I2C_MDR_DLB BSP_BIT32(6) 217 #define TMS570_I2C_MDR_nIRS BSP_BIT32(5) 220 #define TMS570_I2C_MDR_STB BSP_BIT32(4) 223 #define TMS570_I2C_MDR_FDF BSP_BIT32(3) 226 #define TMS570_I2C_MDR_BC(val) BSP_FLD32(val,0, 2) 227 #define TMS570_I2C_MDR_BC_GET(reg) BSP_FLD32GET(reg,0, 2) 228 #define TMS570_I2C_MDR_BC_SET(reg,val) BSP_FLD32SET(reg, val,0, 2) 233 #define TMS570_I2C_IVR_TESTMD(val) BSP_FLD32(val,8, 11) 234 #define TMS570_I2C_IVR_TESTMD_GET(reg) BSP_FLD32GET(reg,8, 11) 235 #define TMS570_I2C_IVR_TESTMD_SET(reg,val) BSP_FLD32SET(reg, val,8, 11) 238 #define TMS570_I2C_IVR_INTCODE(val) BSP_FLD32(val,0, 2) 239 #define TMS570_I2C_IVR_INTCODE_GET(reg) BSP_FLD32GET(reg,0, 2) 240 #define TMS570_I2C_IVR_INTCODE_SET(reg,val) BSP_FLD32SET(reg, val,0, 2) 245 #define TMS570_I2C_EMDR_IGNACK BSP_BIT32(1) 248 #define TMS570_I2C_EMDR_BCM BSP_BIT32(0) 253 #define TMS570_I2C_PSC_PSC(val) BSP_FLD32(val,0, 7) 254 #define TMS570_I2C_PSC_PSC_GET(reg) BSP_FLD32GET(reg,0, 7) 255 #define TMS570_I2C_PSC_PSC_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 260 #define TMS570_I2C_PID11_CLASS(val) BSP_FLD32(val,8, 15) 261 #define TMS570_I2C_PID11_CLASS_GET(reg) BSP_FLD32GET(reg,8, 15) 262 #define TMS570_I2C_PID11_CLASS_SET(reg,val) BSP_FLD32SET(reg, val,8, 15) 265 #define TMS570_I2C_PID11_REVISION(val) BSP_FLD32(val,0, 7) 266 #define TMS570_I2C_PID11_REVISION_GET(reg) BSP_FLD32GET(reg,0, 7) 267 #define TMS570_I2C_PID11_REVISION_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 272 #define TMS570_I2C_PID12_TYPE(val) BSP_FLD32(val,0, 7) 273 #define TMS570_I2C_PID12_TYPE_GET(reg) BSP_FLD32GET(reg,0, 7) 274 #define TMS570_I2C_PID12_TYPE_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 279 #define TMS570_I2C_DMACR_TXDMAEN BSP_BIT32(1) 282 #define TMS570_I2C_DMACR_RXDMAEN BSP_BIT32(0) 287 #define TMS570_I2C_PFNC_PINFUNC BSP_BIT32(0) 292 #define TMS570_I2C_DIR_SDADIR BSP_BIT32(1) 295 #define TMS570_I2C_DIR_SCLDIR BSP_BIT32(0) 300 #define TMS570_I2C_DIN_SDAIN BSP_BIT32(1) 303 #define TMS570_I2C_DIN_SCLIN BSP_BIT32(0) 308 #define TMS570_I2C_DOUT_SDAOUT BSP_BIT32(1) 311 #define TMS570_I2C_DOUT_SCLOUT BSP_BIT32(0) 316 #define TMS570_I2C_SET_SDASET BSP_BIT32(1) 319 #define TMS570_I2C_SET_SCLSET BSP_BIT32(0) 324 #define TMS570_I2C_CLR_SDACLR BSP_BIT32(1) 327 #define TMS570_I2C_CLR_SCLCLR BSP_BIT32(0) 332 #define TMS570_I2C_PDR_SDAPDR BSP_BIT32(1) 335 #define TMS570_I2C_PDR_SCLPDR BSP_BIT32(0) 340 #define TMS570_I2C_PDIS_SDAPDIS BSP_BIT32(1) 343 #define TMS570_I2C_PDIS_SCLPDIS BSP_BIT32(0) 348 #define TMS570_I2C_PSEL_SDAPSEL BSP_BIT32(1) 351 #define TMS570_I2C_PSEL_SCLPSEL BSP_BIT32(0) 356 #define TMS570_I2C_pSRS_SDASRS BSP_BIT32(1) 359 #define TMS570_I2C_pSRS_SCLSRS BSP_BIT32(0)
#define IMR
interrupt mask reg
Definition: wd80x3.h:103