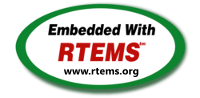 |
RTEMS
5.1
|
Go to the documentation of this file. 31 void BSP_uart_init(
int baud);
35 #define LM32_UART_RBR (0x0000) 39 #define LM32_UART_IER (0x0004) 40 #define LM32_UART_IER_RBRI (0x0001) 41 #define LM32_UART_IER_THRI (0x0002) 42 #define LM32_UART_IER_RLSI (0x0004) 43 #define LM32_UART_IER_MSI (0x0008) 47 #define LM32_UART_IIR (0x0008) 48 #define LM32_UART_IIR_STAT (0x0001) 49 #define LM32_UART_IIR_ID0 (0x0002) 50 #define LM32_UART_IIR_ID1 (0x0004) 54 #define LM32_UART_LCR (0x000C) 55 #define LM32_UART_LCR_WLS0 (0x0001) 56 #define LM32_UART_LCR_WLS1 (0x0002) 57 #define LM32_UART_LCR_STB (0x0004) 58 #define LM32_UART_LCR_PEN (0x0008) 59 #define LM32_UART_LCR_EPS (0x0010) 60 #define LM32_UART_LCR_SP (0x0020) 61 #define LM32_UART_LCR_SB (0x0040) 62 #define LM32_UART_LCR_5BIT (0) 63 #define LM32_UART_LCR_6BIT (LM32_UART_LCR_WLS0) 64 #define LM32_UART_LCR_7BIT (LM32_UART_LCR_WLS1) 65 #define LM32_UART_LCR_8BIT (LM32_UART_LCR_WLS1 | LM32_UART_LCR_WLS0) 69 #define LM32_UART_MCR (0x0010) 70 #define LM32_UART_MCR_DTR (0x0001) 71 #define LM32_UART_MCR_RTS (0x0002) 75 #define LM32_UART_LSR (0x0014) 76 #define LM32_UART_LSR_DR (0x0001) 77 #define LM32_UART_LSR_OE (0x0002) 78 #define LM32_UART_LSR_PE (0x0004) 79 #define LM32_UART_LSR_FE (0x0008) 80 #define LM32_UART_LSR_BI (0x0010) 81 #define LM32_UART_LSR_THRE (0x0020) 82 #define LM32_UART_LSR_TEMT (0x0040) 86 #define LM32_UART_MSR (0x0018) 87 #define LM32_UART_MSR_DCTS (0x0001) 88 #define LM32_UART_MSR_DDSR (0x0002) 89 #define LM32_UART_MSR_TERI (0x0004) 90 #define LM32_UART_MSR_DDCD (0x0008) 91 #define LM32_UART_MSR_CTS (0x0010) 92 #define LM32_UART_MSR_DSR (0x0020) 93 #define LM32_UART_MSR_RI (0x0040) 94 #define LM32_UART_MSR_DCD (0x0000) 98 #define LM32_UART_DIV (0x001C)