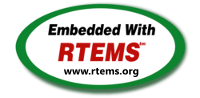 |
RTEMS
5.1
|
29 #define CI_MAGICNUMBER 5 30 #define CI_PCOMPRESSION 7 31 #define CI_ACCOMPRESSION 8 32 #define CI_CALLBACK 13 55 bool neg_pcompression;
56 bool neg_accompression;
72 extern uint32_t xmit_accm[][8];
79 void lcp_close(
int,
char *);
80 void lcp_lowerup(
int);
81 void lcp_lowerdown(
int);
82 void lcp_sprotrej(
int, u_char *,
int);
84 extern struct protent lcp_protent;
88 #define DEFLOOPBACKFAIL 10