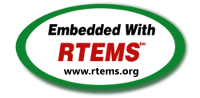 |
RTEMS 6.1-rc6
|
Loading...
Searching...
No Matches
23#define __MFSPR(reg, val) \
24 __asm__ __volatile__("mfspr %0,"#reg : "=r" (val))
26#define __MTSPR(val, reg) \
27 __asm__ __volatile__("mtspr "#reg",%0" : : "r" (val))
31static inline unsigned long _read_##reg(void) \
37static inline void _write_##reg(unsigned long val)\
44static inline unsigned long _read_##reg(void) \
51static inline unsigned long _read_MSR(
void)
54 __asm__
volatile(
"mfmsr %0" :
"=r" (val));
58static inline void _write_MSR(
unsigned long val)
60 __asm__
volatile(
"mtmsr %0" : :
"r" (val));
64static inline unsigned long _read_SR(
void * va)
78static inline void _write_SR(
unsigned long val,
void * va)
84 ".machine \"pop\"" : :
85 "r" (val) ,
"r" (va) :