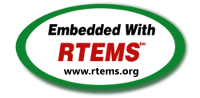 |
RTEMS 6.1-rc6
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
48#ifndef _RTEMS_M68K_SIM_H
49#define _RTEMS_M68K_SIM_H
54#define SAM(a,b,c) ((a << b) & c)
61#define SIM_VOLATILE_USHORT_POINTER
62#define SIM_VOLATILE_UCHAR_POINTER
64#define SIM_VOLATILE_USHORT_POINTER (volatile unsigned short int * const)
65#define SIM_VOLATILE_UCHAR_POINTER (volatile unsigned char * const)
72#define SIM_CRB 0x7ffa00
76#define SIM_CRB 0xfffa00
81#define SIMCR SIM_VOLATILE_USHORT_POINTER(0x00 + SIM_CRB)
94#define SIMTR SIM_VOLATILE_USHORT_POINTER(0x02 + SIM_CRB)
100#define SYNCR SIM_VOLATILE_USHORT_POINTER(0x04 + SIM_CRB)
103#define PRESCALE 0x4000
104#define COUNTER 0x3f00
114#define RSR SIM_VOLATILE_UCHAR_POINTER(0x07 + SIM_CRB)
126#define SIMTRE SIM_VOLATILE_USHORT_POINTER(0x08 + SIM_CRB)
132#define PORTE0 SIM_VOLATILE_UCHAR_POINTER(0x11 + SIM_CRB)
133#define PORTE1 SIM_VOLATILE_UCHAR_POINTER(0x13 + SIM_CRB)
135#define DDRE SIM_VOLATILE_UCHAR_POINTER(0x15 + SIM_CRB)
137#define PEPAR SIM_VOLATILE_UCHAR_POINTER(0x17 + SIM_CRB)
145#define PORTF0 SIM_VOLATILE_UCHAR_POINTER(0x19 + SIM_CRB)
146#define PORTF1 SIM_VOLATILE_UCHAR_POINTER(0x1b + SIM_CRB)
148#define DDRF SIM_VOLATILE_UCHAR_POINTER(0x1d + SIM_CRB)
150#define PFPAR SIM_VOLATILE_UCHAR_POINTER(0x1f + SIM_CRB)
157#define SYPCR SIM_VOLATILE_UCHAR_POINTER(0x21 + SIM_CRB)
169#define PICR SIM_VOLATILE_USHORT_POINTER(0x22 + SIM_CRB)
176#define PITR SIM_VOLATILE_USHORT_POINTER(0x24 + SIM_CRB)
183#define SWSR SIM_VOLATILE_UCHAR_POINTER(0x27 + SIM_CRB)
189#define TSTMSRA SIM_VOLATILE_USHORT_POINTER(0x30 + SIM_CRB)
191#define TSTMSRB SIM_VOLATILE_USHORT_POINTER(0x32 + SIM_CRB)
193#define TSTSC SIM_VOLATILE_USHORT_POINTER(0x34 + SIM_CRB)
195#define TSTRC SIM_VOLATILE_USHORT_POINTER(0x36 + SIM_CRB)
197#define CREG SIM_VOLATILE_USHORT_POINTER(0x38 + SIM_CRB)
199#define DREG SIM_VOLATILE_USHORT_POINTER(0x3a + SIM_CRB)
205#define PORTC SIM_VOLATILE_UCHAR_POINTER(0x41 + SIM_CRB)
210#define CSPAR0 SIM_VOLATILE_USHORT_POINTER(0x44 + SIM_CRB)
217#define CSPAR1 SIM_VOLATILE_USHORT_POINTER(0x46 + SIM_CRB)
263#define CSBARBT SIM_VOLATILE_USHORT_POINTER(0x48 + SIM_CRB)
264#define CSBAR0 SIM_VOLATILE_USHORT_POINTER(0x4c + SIM_CRB)
265#define CSBAR1 SIM_VOLATILE_USHORT_POINTER(0x50 + SIM_CRB)
266#define CSBAR2 SIM_VOLATILE_USHORT_POINTER(0x54 + SIM_CRB)
267#define CSBAR3 SIM_VOLATILE_USHORT_POINTER(0x58 + SIM_CRB)
268#define CSBAR4 SIM_VOLATILE_USHORT_POINTER(0x5c + SIM_CRB)
269#define CSBAR5 SIM_VOLATILE_USHORT_POINTER(0x60 + SIM_CRB)
270#define CSBAR6 SIM_VOLATILE_USHORT_POINTER(0x64 + SIM_CRB)
271#define CSBAR7 SIM_VOLATILE_USHORT_POINTER(0x68 + SIM_CRB)
272#define CSBAR8 SIM_VOLATILE_USHORT_POINTER(0x6c + SIM_CRB)
273#define CSBAR9 SIM_VOLATILE_USHORT_POINTER(0x70 + SIM_CRB)
274#define CSBAR10 SIM_VOLATILE_USHORT_POINTER(0x74 + SIM_CRB)
278#define LowerByte 0x2000
279#define UpperByte 0x4000
280#define BothBytes 0x6000
281#define ReadOnly 0x0800
282#define WriteOnly 0x1000
283#define ReadWrite 0x1800
287#define WaitStates_0 (0x0 << 6)
288#define WaitStates_1 (0x1 << 6)
289#define WaitStates_2 (0x2 << 6)
290#define WaitStates_3 (0x3 << 6)
291#define WaitStates_4 (0x4 << 6)
292#define WaitStates_5 (0x5 << 6)
293#define WaitStates_6 (0x6 << 6)
294#define WaitStates_7 (0x7 << 6)
295#define WaitStates_8 (0x8 << 6)
296#define WaitStates_9 (0x9 << 6)
297#define WaitStates_10 (0xa << 6)
298#define WaitStates_11 (0xb << 6)
299#define WaitStates_12 (0xc << 6)
300#define WaitStates_13 (0xd << 6)
301#define FastTerm (0xe << 6)
302#define External (0xf << 6)
304#define CPUSpace (0x0 << 4)
305#define UserSpace (0x1 << 4)
306#define SupSpace (0x2 << 4)
307#define UserSupSpace (0x3 << 4)
309#define IPLevel_any 0x0
320#define CSORBT SIM_VOLATILE_USHORT_POINTER(0x4a + SIM_CRB)
321#define CSOR0 SIM_VOLATILE_USHORT_POINTER(0x4e + SIM_CRB)
322#define CSOR1 SIM_VOLATILE_USHORT_POINTER(0x52 + SIM_CRB)
323#define CSOR2 SIM_VOLATILE_USHORT_POINTER(0x56 + SIM_CRB)
324#define CSOR3 SIM_VOLATILE_USHORT_POINTER(0x5a + SIM_CRB)
325#define CSOR4 SIM_VOLATILE_USHORT_POINTER(0x5e + SIM_CRB)
326#define CSOR5 SIM_VOLATILE_USHORT_POINTER(0x62 + SIM_CRB)
327#define CSOR6 SIM_VOLATILE_USHORT_POINTER(0x66 + SIM_CRB)
328#define CSOR7 SIM_VOLATILE_USHORT_POINTER(0x6a + SIM_CRB)
329#define CSOR8 SIM_VOLATILE_USHORT_POINTER(0x6e + SIM_CRB)
330#define CSOR9 SIM_VOLATILE_USHORT_POINTER(0x72 + SIM_CRB)
331#define CSOR10 SIM_VOLATILE_USHORT_POINTER(0x76 + SIM_CRB)