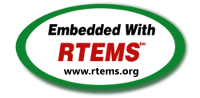 |
RTEMS 6.1-rc6
|
Loading...
Searching...
No Matches
16#ifndef _LIBCPU_PGTABLE_H
17#define _LIBCPU_PGTABLE_H
52#define PMD_SIZE (1UL << PMD_SHIFT)
53#define PMD_MASK (~(PMD_SIZE-1))
57#define PGDIR_SIZE (1UL << PGDIR_SHIFT)
58#define PGDIR_MASK (~(PGDIR_SIZE-1))
64#define PTRS_PER_PTE 1024
66#define PTRS_PER_PGD 1024
67#define USER_PTRS_PER_PGD (TASK_SIZE / PGDIR_SIZE)
85#define VMALLOC_OFFSET (0x4000000)
86#define VMALLOC_START ((((long)high_memory + VMALLOC_OFFSET) & ~(VMALLOC_OFFSET-1)))
87#define VMALLOC_VMADDR(x) ((unsigned long)(x))
88#define VMALLOC_END ioremap_bot
94#define _PAGE_PRESENT 0x001
95#define _PAGE_USER 0x002
97#define _PAGE_GUARDED 0x008
98#define _PAGE_COHERENT 0x010
99#define _PAGE_NO_CACHE 0x020
100#define _PAGE_WRITETHRU 0x040
101#define _PAGE_DIRTY 0x080
102#define _PAGE_ACCESSED 0x100
103#define _PAGE_HWWRITE 0x200
104#define _PAGE_SHARED 0
106#define _PAGE_CHG_MASK (PAGE_MASK | _PAGE_ACCESSED | _PAGE_DIRTY)
108#define _PAGE_BASE _PAGE_PRESENT | _PAGE_ACCESSED
109#define _PAGE_WRENABLE _PAGE_RW | _PAGE_DIRTY | _PAGE_HWWRITE
111#define PAGE_NONE __pgprot(_PAGE_PRESENT | _PAGE_ACCESSED)
113#define PAGE_SHARED __pgprot(_PAGE_BASE | _PAGE_RW | _PAGE_USER | \
115#define PAGE_COPY __pgprot(_PAGE_BASE | _PAGE_USER)
116#define PAGE_READONLY __pgprot(_PAGE_BASE | _PAGE_USER)
117#define PAGE_KERNEL __pgprot(_PAGE_BASE | _PAGE_WRENABLE | _PAGE_SHARED)
118#define PAGE_KERNEL_CI __pgprot(_PAGE_BASE | _PAGE_WRENABLE | _PAGE_SHARED | \
127#define __P000 PAGE_NONE
128#define __P001 PAGE_READONLY
129#define __P010 PAGE_COPY
130#define __P011 PAGE_COPY
131#define __P100 PAGE_READONLY
132#define __P101 PAGE_READONLY
133#define __P110 PAGE_COPY
134#define __P111 PAGE_COPY
136#define __S000 PAGE_NONE
137#define __S001 PAGE_READONLY
138#define __S010 PAGE_SHARED
139#define __S011 PAGE_SHARED
140#define __S100 PAGE_READONLY
141#define __S101 PAGE_READONLY
142#define __S110 PAGE_SHARED
143#define __S111 PAGE_SHARED