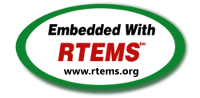 |
RTEMS 6.1-rc6
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
47#ifndef _RTEMS_SCORE_BSD_TREE_H_
48#define _RTEMS_SCORE_BSD_TREE_H_
79#define RTEMS_SPLAY_HEAD(name, type) \
81 struct type *sph_root; \
84#define RTEMS_SPLAY_INITIALIZER(root) \
87#define RTEMS_SPLAY_INIT(root) do { \
88 (root)->sph_root = NULL; \
91#define RTEMS_SPLAY_ENTRY(type) \
93 struct type *spe_left; \
94 struct type *spe_right; \
97#define RTEMS_SPLAY_LEFT(elm, field) (elm)->field.spe_left
98#define RTEMS_SPLAY_RIGHT(elm, field) (elm)->field.spe_right
99#define RTEMS_SPLAY_ROOT(head) (head)->sph_root
100#define RTEMS_SPLAY_EMPTY(head) (RTEMS_SPLAY_ROOT(head) == NULL)
103#define RTEMS_SPLAY_ROTATE_RIGHT(head, tmp, field) do { \
104 RTEMS_SPLAY_LEFT((head)->sph_root, field) = RTEMS_SPLAY_RIGHT(tmp, field); \
105 RTEMS_SPLAY_RIGHT(tmp, field) = (head)->sph_root; \
106 (head)->sph_root = tmp; \
109#define RTEMS_SPLAY_ROTATE_LEFT(head, tmp, field) do { \
110 RTEMS_SPLAY_RIGHT((head)->sph_root, field) = RTEMS_SPLAY_LEFT(tmp, field); \
111 RTEMS_SPLAY_LEFT(tmp, field) = (head)->sph_root; \
112 (head)->sph_root = tmp; \
115#define RTEMS_SPLAY_LINKLEFT(head, tmp, field) do { \
116 RTEMS_SPLAY_LEFT(tmp, field) = (head)->sph_root; \
117 tmp = (head)->sph_root; \
118 (head)->sph_root = RTEMS_SPLAY_LEFT((head)->sph_root, field); \
121#define RTEMS_SPLAY_LINKRIGHT(head, tmp, field) do { \
122 RTEMS_SPLAY_RIGHT(tmp, field) = (head)->sph_root; \
123 tmp = (head)->sph_root; \
124 (head)->sph_root = RTEMS_SPLAY_RIGHT((head)->sph_root, field); \
127#define RTEMS_SPLAY_ASSEMBLE(head, node, left, right, field) do { \
128 RTEMS_SPLAY_RIGHT(left, field) = RTEMS_SPLAY_LEFT((head)->sph_root, field); \
129 RTEMS_SPLAY_LEFT(right, field) = RTEMS_SPLAY_RIGHT((head)->sph_root, field);\
130 RTEMS_SPLAY_LEFT((head)->sph_root, field) = RTEMS_SPLAY_RIGHT(node, field); \
131 RTEMS_SPLAY_RIGHT((head)->sph_root, field) = RTEMS_SPLAY_LEFT(node, field); \
136#define RTEMS_SPLAY_PROTOTYPE(name, type, field, cmp) \
137void name##_SPLAY(struct name *, struct type *); \
138void name##_RTEMS_SPLAY_MINMAX(struct name *, int); \
139struct type *name##_RTEMS_SPLAY_INSERT(struct name *, struct type *); \
140struct type *name##_RTEMS_SPLAY_REMOVE(struct name *, struct type *); \
143static __unused __inline struct type * \
144name##_RTEMS_SPLAY_FIND(struct name *head, struct type *elm) \
146 if (RTEMS_SPLAY_EMPTY(head)) \
148 name##_SPLAY(head, elm); \
149 if ((cmp)(elm, (head)->sph_root) == 0) \
150 return (head->sph_root); \
154static __unused __inline struct type * \
155name##_RTEMS_SPLAY_NEXT(struct name *head, struct type *elm) \
157 name##_SPLAY(head, elm); \
158 if (RTEMS_SPLAY_RIGHT(elm, field) != NULL) { \
159 elm = RTEMS_SPLAY_RIGHT(elm, field); \
160 while (RTEMS_SPLAY_LEFT(elm, field) != NULL) { \
161 elm = RTEMS_SPLAY_LEFT(elm, field); \
168static __unused __inline struct type * \
169name##_RTEMS_SPLAY_MIN_MAX(struct name *head, int val) \
171 name##_RTEMS_SPLAY_MINMAX(head, val); \
172 return (RTEMS_SPLAY_ROOT(head)); \
178#define RTEMS_SPLAY_GENERATE(name, type, field, cmp) \
180name##_RTEMS_SPLAY_INSERT(struct name *head, struct type *elm) \
182 if (RTEMS_SPLAY_EMPTY(head)) { \
183 RTEMS_SPLAY_LEFT(elm, field) = RTEMS_SPLAY_RIGHT(elm, field) = NULL; \
186 name##_SPLAY(head, elm); \
187 __comp = (cmp)(elm, (head)->sph_root); \
189 RTEMS_SPLAY_LEFT(elm, field) = RTEMS_SPLAY_LEFT((head)->sph_root, field);\
190 RTEMS_SPLAY_RIGHT(elm, field) = (head)->sph_root; \
191 RTEMS_SPLAY_LEFT((head)->sph_root, field) = NULL; \
192 } else if (__comp > 0) { \
193 RTEMS_SPLAY_RIGHT(elm, field) = RTEMS_SPLAY_RIGHT((head)->sph_root, field);\
194 RTEMS_SPLAY_LEFT(elm, field) = (head)->sph_root; \
195 RTEMS_SPLAY_RIGHT((head)->sph_root, field) = NULL; \
197 return ((head)->sph_root); \
199 (head)->sph_root = (elm); \
204name##_RTEMS_SPLAY_REMOVE(struct name *head, struct type *elm) \
206 struct type *__tmp; \
207 if (RTEMS_SPLAY_EMPTY(head)) \
209 name##_SPLAY(head, elm); \
210 if ((cmp)(elm, (head)->sph_root) == 0) { \
211 if (RTEMS_SPLAY_LEFT((head)->sph_root, field) == NULL) { \
212 (head)->sph_root = RTEMS_SPLAY_RIGHT((head)->sph_root, field);\
214 __tmp = RTEMS_SPLAY_RIGHT((head)->sph_root, field); \
215 (head)->sph_root = RTEMS_SPLAY_LEFT((head)->sph_root, field);\
216 name##_SPLAY(head, elm); \
217 RTEMS_SPLAY_RIGHT((head)->sph_root, field) = __tmp; \
225name##_SPLAY(struct name *head, struct type *elm) \
227 struct type __node, *__left, *__right, *__tmp; \
230 RTEMS_SPLAY_LEFT(&__node, field) = RTEMS_SPLAY_RIGHT(&__node, field) = NULL;\
231 __left = __right = &__node; \
233 while ((__comp = (cmp)(elm, (head)->sph_root)) != 0) { \
235 __tmp = RTEMS_SPLAY_LEFT((head)->sph_root, field); \
238 if ((cmp)(elm, __tmp) < 0){ \
239 RTEMS_SPLAY_ROTATE_RIGHT(head, __tmp, field); \
240 if (RTEMS_SPLAY_LEFT((head)->sph_root, field) == NULL)\
243 RTEMS_SPLAY_LINKLEFT(head, __right, field); \
244 } else if (__comp > 0) { \
245 __tmp = RTEMS_SPLAY_RIGHT((head)->sph_root, field); \
248 if ((cmp)(elm, __tmp) > 0){ \
249 RTEMS_SPLAY_ROTATE_LEFT(head, __tmp, field); \
250 if (RTEMS_SPLAY_RIGHT((head)->sph_root, field) == NULL)\
253 RTEMS_SPLAY_LINKRIGHT(head, __left, field); \
256 RTEMS_SPLAY_ASSEMBLE(head, &__node, __left, __right, field); \
262void name##_RTEMS_SPLAY_MINMAX(struct name *head, int __comp) \
264 struct type __node, *__left, *__right, *__tmp; \
266 RTEMS_SPLAY_LEFT(&__node, field) = RTEMS_SPLAY_RIGHT(&__node, field) = NULL;\
267 __left = __right = &__node; \
271 __tmp = RTEMS_SPLAY_LEFT((head)->sph_root, field); \
275 RTEMS_SPLAY_ROTATE_RIGHT(head, __tmp, field); \
276 if (RTEMS_SPLAY_LEFT((head)->sph_root, field) == NULL)\
279 RTEMS_SPLAY_LINKLEFT(head, __right, field); \
280 } else if (__comp > 0) { \
281 __tmp = RTEMS_SPLAY_RIGHT((head)->sph_root, field); \
285 RTEMS_SPLAY_ROTATE_LEFT(head, __tmp, field); \
286 if (RTEMS_SPLAY_RIGHT((head)->sph_root, field) == NULL)\
289 RTEMS_SPLAY_LINKRIGHT(head, __left, field); \
292 RTEMS_SPLAY_ASSEMBLE(head, &__node, __left, __right, field); \
295#define RTEMS_SPLAY_NEGINF -1
296#define RTEMS_SPLAY_INF 1
298#define RTEMS_SPLAY_INSERT(name, x, y) name##_RTEMS_SPLAY_INSERT(x, y)
299#define RTEMS_SPLAY_REMOVE(name, x, y) name##_RTEMS_SPLAY_REMOVE(x, y)
300#define RTEMS_SPLAY_FIND(name, x, y) name##_RTEMS_SPLAY_FIND(x, y)
301#define RTEMS_SPLAY_NEXT(name, x, y) name##_RTEMS_SPLAY_NEXT(x, y)
302#define RTEMS_SPLAY_MIN(name, x) (RTEMS_SPLAY_EMPTY(x) ? NULL \
303 : name##_RTEMS_SPLAY_MIN_MAX(x, RTEMS_SPLAY_NEGINF))
304#define RTEMS_SPLAY_MAX(name, x) (RTEMS_SPLAY_EMPTY(x) ? NULL \
305 : name##_RTEMS_SPLAY_MIN_MAX(x, RTEMS_SPLAY_INF))
307#define RTEMS_SPLAY_FOREACH(x, name, head) \
308 for ((x) = RTEMS_SPLAY_MIN(name, head); \
310 (x) = RTEMS_SPLAY_NEXT(name, head, x))
313#define RTEMS_RB_HEAD(name, type) \
315 struct type *rbh_root; \
318#define RTEMS_RB_INITIALIZER(root) \
321#define RTEMS_RB_INIT(root) do { \
322 (root)->rbh_root = NULL; \
325#define RTEMS_RB_BLACK 0
326#define RTEMS_RB_RED 1
327#define RTEMS_RB_ENTRY(type) \
329 struct type *rbe_left; \
330 struct type *rbe_right; \
331 struct type *rbe_parent; \
335#define RTEMS_RB_LEFT(elm, field) (elm)->field.rbe_left
336#define RTEMS_RB_RIGHT(elm, field) (elm)->field.rbe_right
337#define RTEMS_RB_PARENT(elm, field) (elm)->field.rbe_parent
338#define RTEMS_RB_COLOR(elm, field) (elm)->field.rbe_color
339#define RTEMS_RB_ISRED(elm, field) ((elm) != NULL && RTEMS_RB_COLOR(elm, field) == RTEMS_RB_RED)
340#define RTEMS_RB_ROOT(head) (head)->rbh_root
341#define RTEMS_RB_EMPTY(head) (RTEMS_RB_ROOT(head) == NULL)
343#define RTEMS_RB_SET_PARENT(dst, src, field) do { \
344 RTEMS_RB_PARENT(dst, field) = src; \
347#define RTEMS_RB_SET(elm, parent, field) do { \
348 RTEMS_RB_SET_PARENT(elm, parent, field); \
349 RTEMS_RB_LEFT(elm, field) = RTEMS_RB_RIGHT(elm, field) = NULL; \
350 RTEMS_RB_COLOR(elm, field) = RTEMS_RB_RED; \
353#define RTEMS_RB_SET_BLACKRED(black, red, field) do { \
354 RTEMS_RB_COLOR(black, field) = RTEMS_RB_BLACK; \
355 RTEMS_RB_COLOR(red, field) = RTEMS_RB_RED; \
362#ifndef RTEMS_RB_AUGMENT
363#define RTEMS_RB_AUGMENT(x) break
366#define RTEMS_RB_SWAP_CHILD(head, out, in, field) do { \
367 if (RTEMS_RB_PARENT(out, field) == NULL) \
368 RTEMS_RB_ROOT(head) = (in); \
369 else if ((out) == RTEMS_RB_LEFT(RTEMS_RB_PARENT(out, field), field)) \
370 RTEMS_RB_LEFT(RTEMS_RB_PARENT(out, field), field) = (in); \
372 RTEMS_RB_RIGHT(RTEMS_RB_PARENT(out, field), field) = (in); \
375#define RTEMS_RB_ROTATE_LEFT(head, elm, tmp, field) do { \
376 (tmp) = RTEMS_RB_RIGHT(elm, field); \
377 if ((RTEMS_RB_RIGHT(elm, field) = RTEMS_RB_LEFT(tmp, field)) != NULL) { \
378 RTEMS_RB_SET_PARENT(RTEMS_RB_RIGHT(elm, field), elm, field); \
380 RTEMS_RB_SET_PARENT(tmp, RTEMS_RB_PARENT(elm, field), field); \
381 RTEMS_RB_SWAP_CHILD(head, elm, tmp, field); \
382 RTEMS_RB_LEFT(tmp, field) = (elm); \
383 RTEMS_RB_SET_PARENT(elm, tmp, field); \
384 RTEMS_RB_AUGMENT(elm); \
387#define RTEMS_RB_ROTATE_RIGHT(head, elm, tmp, field) do { \
388 (tmp) = RTEMS_RB_LEFT(elm, field); \
389 if ((RTEMS_RB_LEFT(elm, field) = RTEMS_RB_RIGHT(tmp, field)) != NULL) { \
390 RTEMS_RB_SET_PARENT(RTEMS_RB_LEFT(elm, field), elm, field); \
392 RTEMS_RB_SET_PARENT(tmp, RTEMS_RB_PARENT(elm, field), field); \
393 RTEMS_RB_SWAP_CHILD(head, elm, tmp, field); \
394 RTEMS_RB_RIGHT(tmp, field) = (elm); \
395 RTEMS_RB_SET_PARENT(elm, tmp, field); \
396 RTEMS_RB_AUGMENT(elm); \
406#define RTEMS_RB_PARENT_ROTATE_LEFT(parent, left, tmp, field) do { \
407 (tmp) = RTEMS_RB_RIGHT(left, field); \
408 if ((RTEMS_RB_RIGHT(left, field) = RTEMS_RB_LEFT(tmp, field)) != NULL) { \
409 RTEMS_RB_SET_PARENT(RTEMS_RB_RIGHT(left, field), left, field); \
411 RTEMS_RB_SET_PARENT(tmp, parent, field); \
412 RTEMS_RB_LEFT(parent, field) = (tmp); \
413 RTEMS_RB_LEFT(tmp, field) = (left); \
414 RTEMS_RB_SET_PARENT(left, tmp, field); \
415 RTEMS_RB_AUGMENT(left); \
418#define RTEMS_RB_PARENT_ROTATE_RIGHT(parent, right, tmp, field) do { \
419 (tmp) = RTEMS_RB_LEFT(right, field); \
420 if ((RTEMS_RB_LEFT(right, field) = RTEMS_RB_RIGHT(tmp, field)) != NULL) { \
421 RTEMS_RB_SET_PARENT(RTEMS_RB_LEFT(right, field), right, field); \
423 RTEMS_RB_SET_PARENT(tmp, parent, field); \
424 RTEMS_RB_RIGHT(parent, field) = (tmp); \
425 RTEMS_RB_RIGHT(tmp, field) = (right); \
426 RTEMS_RB_SET_PARENT(right, tmp, field); \
427 RTEMS_RB_AUGMENT(right); \
447#define RTEMS_RB_RED_ROTATE_LEFT(head, elm, tmp, field) do { \
448 (tmp) = RTEMS_RB_RIGHT(elm, field); \
449 RTEMS_RB_RIGHT(elm, field) = RTEMS_RB_LEFT(tmp, field); \
450 RTEMS_RB_SET_PARENT(RTEMS_RB_RIGHT(elm, field), elm, field); \
451 RTEMS_RB_SET_PARENT(tmp, RTEMS_RB_PARENT(elm, field), field); \
452 RTEMS_RB_SWAP_CHILD(head, elm, tmp, field); \
453 RTEMS_RB_LEFT(tmp, field) = (elm); \
454 RTEMS_RB_SET_PARENT(elm, tmp, field); \
455 RTEMS_RB_AUGMENT(elm); \
458#define RTEMS_RB_RED_ROTATE_RIGHT(head, elm, tmp, field) do { \
459 (tmp) = RTEMS_RB_LEFT(elm, field); \
460 RTEMS_RB_LEFT(elm, field) = RTEMS_RB_RIGHT(tmp, field); \
461 RTEMS_RB_SET_PARENT(RTEMS_RB_LEFT(elm, field), elm, field); \
462 RTEMS_RB_SET_PARENT(tmp, RTEMS_RB_PARENT(elm, field), field); \
463 RTEMS_RB_SWAP_CHILD(head, elm, tmp, field); \
464 RTEMS_RB_RIGHT(tmp, field) = (elm); \
465 RTEMS_RB_SET_PARENT(elm, tmp, field); \
466 RTEMS_RB_AUGMENT(elm); \
470#define RTEMS_RB_PROTOTYPE(name, type, field, cmp) \
471 RTEMS_RB_PROTOTYPE_INTERNAL(name, type, field, cmp,)
472#define RTEMS_RB_PROTOTYPE_STATIC(name, type, field, cmp) \
473 RTEMS_RB_PROTOTYPE_INTERNAL(name, type, field, cmp, __unused static)
474#define RTEMS_RB_PROTOTYPE_INTERNAL(name, type, field, cmp, attr) \
475 RTEMS_RB_PROTOTYPE_INSERT_COLOR(name, type, attr); \
476 RTEMS_RB_PROTOTYPE_REMOVE_COLOR(name, type, attr); \
477 RTEMS_RB_PROTOTYPE_INSERT(name, type, attr); \
478 RTEMS_RB_PROTOTYPE_REMOVE(name, type, attr); \
479 RTEMS_RB_PROTOTYPE_FIND(name, type, attr); \
480 RTEMS_RB_PROTOTYPE_NFIND(name, type, attr); \
481 RTEMS_RB_PROTOTYPE_NEXT(name, type, attr); \
482 RTEMS_RB_PROTOTYPE_PREV(name, type, attr); \
483 RTEMS_RB_PROTOTYPE_MINMAX(name, type, attr); \
484 RTEMS_RB_PROTOTYPE_REINSERT(name, type, attr);
485#define RTEMS_RB_PROTOTYPE_INSERT_COLOR(name, type, attr) \
486 attr void name##_RTEMS_RB_INSERT_COLOR(struct name *, struct type *)
487#define RTEMS_RB_PROTOTYPE_REMOVE_COLOR(name, type, attr) \
488 attr void name##_RTEMS_RB_REMOVE_COLOR(struct name *, struct type *)
489#define RTEMS_RB_PROTOTYPE_REMOVE(name, type, attr) \
490 attr struct type *name##_RTEMS_RB_REMOVE(struct name *, struct type *)
491#define RTEMS_RB_PROTOTYPE_INSERT(name, type, attr) \
492 attr struct type *name##_RTEMS_RB_INSERT(struct name *, struct type *)
493#define RTEMS_RB_PROTOTYPE_FIND(name, type, attr) \
494 attr struct type *name##_RTEMS_RB_FIND(struct name *, struct type *)
495#define RTEMS_RB_PROTOTYPE_NFIND(name, type, attr) \
496 attr struct type *name##_RTEMS_RB_NFIND(struct name *, struct type *)
497#define RTEMS_RB_PROTOTYPE_NEXT(name, type, attr) \
498 attr struct type *name##_RTEMS_RB_NEXT(struct type *)
499#define RTEMS_RB_PROTOTYPE_PREV(name, type, attr) \
500 attr struct type *name##_RTEMS_RB_PREV(struct type *)
501#define RTEMS_RB_PROTOTYPE_MINMAX(name, type, attr) \
502 attr struct type *name##_RTEMS_RB_MINMAX(struct name *, int)
503#define RTEMS_RB_PROTOTYPE_REINSERT(name, type, attr) \
504 attr struct type *name##_RTEMS_RB_REINSERT(struct name *, struct type *)
509#define RTEMS_RB_GENERATE(name, type, field, cmp) \
510 RTEMS_RB_GENERATE_INTERNAL(name, type, field, cmp,)
511#define RTEMS_RB_GENERATE_STATIC(name, type, field, cmp) \
512 RTEMS_RB_GENERATE_INTERNAL(name, type, field, cmp, __unused static)
513#define RTEMS_RB_GENERATE_INTERNAL(name, type, field, cmp, attr) \
514 RTEMS_RB_GENERATE_INSERT_COLOR(name, type, field, attr) \
515 RTEMS_RB_GENERATE_REMOVE_COLOR(name, type, field, attr) \
516 RTEMS_RB_GENERATE_INSERT(name, type, field, cmp, attr) \
517 RTEMS_RB_GENERATE_REMOVE(name, type, field, attr) \
518 RTEMS_RB_GENERATE_FIND(name, type, field, cmp, attr) \
519 RTEMS_RB_GENERATE_NFIND(name, type, field, cmp, attr) \
520 RTEMS_RB_GENERATE_NEXT(name, type, field, attr) \
521 RTEMS_RB_GENERATE_PREV(name, type, field, attr) \
522 RTEMS_RB_GENERATE_MINMAX(name, type, field, attr) \
523 RTEMS_RB_GENERATE_REINSERT(name, type, field, cmp, attr)
526#define RTEMS_RB_GENERATE_INSERT_COLOR(name, type, field, attr) \
528name##_RTEMS_RB_INSERT_COLOR(struct name *head, struct type *elm) \
530 struct type *parent, *gparent, *tmp; \
531 while (RTEMS_RB_ISRED((parent = RTEMS_RB_PARENT(elm, field)), field)) { \
532 gparent = RTEMS_RB_PARENT(parent, field); \
533 if (parent == RTEMS_RB_LEFT(gparent, field)) { \
534 tmp = RTEMS_RB_RIGHT(gparent, field); \
535 if (RTEMS_RB_ISRED(tmp, field)) { \
536 RTEMS_RB_COLOR(tmp, field) = RTEMS_RB_BLACK; \
537 RTEMS_RB_SET_BLACKRED(parent, gparent, field);\
541 if (RTEMS_RB_RIGHT(parent, field) == elm) { \
542 RTEMS_RB_PARENT_ROTATE_LEFT(gparent, parent, \
548 RTEMS_RB_SET_BLACKRED(parent, gparent, field); \
549 RTEMS_RB_ROTATE_RIGHT(head, gparent, tmp, field); \
551 tmp = RTEMS_RB_LEFT(gparent, field); \
552 if (RTEMS_RB_ISRED(tmp, field)) { \
553 RTEMS_RB_COLOR(tmp, field) = RTEMS_RB_BLACK; \
554 RTEMS_RB_SET_BLACKRED(parent, gparent, field);\
558 if (RTEMS_RB_LEFT(parent, field) == elm) { \
559 RTEMS_RB_PARENT_ROTATE_RIGHT(gparent, parent, \
565 RTEMS_RB_SET_BLACKRED(parent, gparent, field); \
566 RTEMS_RB_ROTATE_LEFT(head, gparent, tmp, field); \
569 RTEMS_RB_COLOR(head->rbh_root, field) = RTEMS_RB_BLACK; \
572#define RTEMS_RB_GENERATE_REMOVE_COLOR(name, type, field, attr) \
574name##_RTEMS_RB_REMOVE_COLOR(struct name *head, struct type *parent) \
576 struct type *elm, *tmp; \
579 if (RTEMS_RB_LEFT(parent, field) == elm) { \
580 tmp = RTEMS_RB_RIGHT(parent, field); \
581 if (RTEMS_RB_COLOR(tmp, field) == RTEMS_RB_RED) { \
582 RTEMS_RB_SET_BLACKRED(tmp, parent, field); \
583 RTEMS_RB_RED_ROTATE_LEFT(head, parent, tmp, field); \
584 tmp = RTEMS_RB_RIGHT(parent, field); \
586 if (RTEMS_RB_ISRED(RTEMS_RB_RIGHT(tmp, field), field)) \
587 RTEMS_RB_COLOR(RTEMS_RB_RIGHT(tmp, field), field) = RTEMS_RB_BLACK; \
588 else if (RTEMS_RB_ISRED(RTEMS_RB_LEFT(tmp, field), field)) { \
589 struct type *oleft; \
590 RTEMS_RB_PARENT_ROTATE_RIGHT(parent, tmp, \
592 RTEMS_RB_COLOR(oleft, field) = RTEMS_RB_BLACK; \
595 RTEMS_RB_COLOR(tmp, field) = RTEMS_RB_RED; \
597 parent = RTEMS_RB_PARENT(elm, field); \
600 RTEMS_RB_COLOR(tmp, field) = RTEMS_RB_COLOR(parent, field); \
601 RTEMS_RB_COLOR(parent, field) = RTEMS_RB_BLACK; \
602 RTEMS_RB_ROTATE_LEFT(head, parent, tmp, field); \
603 elm = RTEMS_RB_ROOT(head); \
606 tmp = RTEMS_RB_LEFT(parent, field); \
607 if (RTEMS_RB_COLOR(tmp, field) == RTEMS_RB_RED) { \
608 RTEMS_RB_SET_BLACKRED(tmp, parent, field); \
609 RTEMS_RB_RED_ROTATE_RIGHT(head, parent, tmp, field); \
610 tmp = RTEMS_RB_LEFT(parent, field); \
612 if (RTEMS_RB_ISRED(RTEMS_RB_LEFT(tmp, field), field)) \
613 RTEMS_RB_COLOR(RTEMS_RB_LEFT(tmp, field), field) = RTEMS_RB_BLACK; \
614 else if (RTEMS_RB_ISRED(RTEMS_RB_RIGHT(tmp, field), field)) { \
615 struct type *oright; \
616 RTEMS_RB_PARENT_ROTATE_LEFT(parent, tmp, \
618 RTEMS_RB_COLOR(oright, field) = RTEMS_RB_BLACK; \
621 RTEMS_RB_COLOR(tmp, field) = RTEMS_RB_RED; \
623 parent = RTEMS_RB_PARENT(elm, field); \
626 RTEMS_RB_COLOR(tmp, field) = RTEMS_RB_COLOR(parent, field); \
627 RTEMS_RB_COLOR(parent, field) = RTEMS_RB_BLACK; \
628 RTEMS_RB_ROTATE_RIGHT(head, parent, tmp, field); \
629 elm = RTEMS_RB_ROOT(head); \
632 } while (RTEMS_RB_COLOR(elm, field) == RTEMS_RB_BLACK && parent != NULL); \
633 RTEMS_RB_COLOR(elm, field) = RTEMS_RB_BLACK; \
636#define RTEMS_RB_GENERATE_REMOVE(name, type, field, attr) \
638name##_RTEMS_RB_REMOVE(struct name *head, struct type *elm) \
640 struct type *child, *old, *parent, *right; \
644 parent = RTEMS_RB_PARENT(elm, field); \
645 right = RTEMS_RB_RIGHT(elm, field); \
646 color = RTEMS_RB_COLOR(elm, field); \
647 if (RTEMS_RB_LEFT(elm, field) == NULL) \
648 elm = child = right; \
649 else if (right == NULL) \
650 elm = child = RTEMS_RB_LEFT(elm, field); \
652 if ((child = RTEMS_RB_LEFT(right, field)) == NULL) { \
653 child = RTEMS_RB_RIGHT(right, field); \
654 RTEMS_RB_RIGHT(old, field) = child; \
655 parent = elm = right; \
659 while ((child = RTEMS_RB_LEFT(elm, field)) != NULL); \
660 child = RTEMS_RB_RIGHT(elm, field); \
661 parent = RTEMS_RB_PARENT(elm, field); \
662 RTEMS_RB_LEFT(parent, field) = child; \
663 RTEMS_RB_SET_PARENT(RTEMS_RB_RIGHT(old, field), elm, field); \
665 RTEMS_RB_SET_PARENT(RTEMS_RB_LEFT(old, field), elm, field); \
666 color = RTEMS_RB_COLOR(elm, field); \
667 elm->field = old->field; \
669 RTEMS_RB_SWAP_CHILD(head, old, elm, field); \
670 if (child != NULL) { \
671 RTEMS_RB_SET_PARENT(child, parent, field); \
672 RTEMS_RB_COLOR(child, field) = RTEMS_RB_BLACK; \
673 } else if (color != RTEMS_RB_RED && parent != NULL) \
674 name##_RTEMS_RB_REMOVE_COLOR(head, parent); \
675 while (parent != NULL) { \
676 RTEMS_RB_AUGMENT(parent); \
677 parent = RTEMS_RB_PARENT(parent, field); \
682#define RTEMS_RB_GENERATE_INSERT(name, type, field, cmp, attr) \
685name##_RTEMS_RB_INSERT(struct name *head, struct type *elm) \
688 struct type *parent = NULL; \
690 tmp = RTEMS_RB_ROOT(head); \
693 comp = (cmp)(elm, parent); \
695 tmp = RTEMS_RB_LEFT(tmp, field); \
697 tmp = RTEMS_RB_RIGHT(tmp, field); \
701 RTEMS_RB_SET(elm, parent, field); \
702 if (parent != NULL) { \
704 RTEMS_RB_LEFT(parent, field) = elm; \
706 RTEMS_RB_RIGHT(parent, field) = elm; \
708 RTEMS_RB_ROOT(head) = elm; \
709 name##_RTEMS_RB_INSERT_COLOR(head, elm); \
710 while (elm != NULL) { \
711 RTEMS_RB_AUGMENT(elm); \
712 elm = RTEMS_RB_PARENT(elm, field); \
717#define RTEMS_RB_GENERATE_FIND(name, type, field, cmp, attr) \
720name##_RTEMS_RB_FIND(struct name *head, struct type *elm) \
722 struct type *tmp = RTEMS_RB_ROOT(head); \
725 comp = cmp(elm, tmp); \
727 tmp = RTEMS_RB_LEFT(tmp, field); \
729 tmp = RTEMS_RB_RIGHT(tmp, field); \
736#define RTEMS_RB_GENERATE_NFIND(name, type, field, cmp, attr) \
739name##_RTEMS_RB_NFIND(struct name *head, struct type *elm) \
741 struct type *tmp = RTEMS_RB_ROOT(head); \
742 struct type *res = NULL; \
745 comp = cmp(elm, tmp); \
748 tmp = RTEMS_RB_LEFT(tmp, field); \
751 tmp = RTEMS_RB_RIGHT(tmp, field); \
758#define RTEMS_RB_GENERATE_NEXT(name, type, field, attr) \
761name##_RTEMS_RB_NEXT(struct type *elm) \
763 if (RTEMS_RB_RIGHT(elm, field)) { \
764 elm = RTEMS_RB_RIGHT(elm, field); \
765 while (RTEMS_RB_LEFT(elm, field)) \
766 elm = RTEMS_RB_LEFT(elm, field); \
768 if (RTEMS_RB_PARENT(elm, field) && \
769 (elm == RTEMS_RB_LEFT(RTEMS_RB_PARENT(elm, field), field))) \
770 elm = RTEMS_RB_PARENT(elm, field); \
772 while (RTEMS_RB_PARENT(elm, field) && \
773 (elm == RTEMS_RB_RIGHT(RTEMS_RB_PARENT(elm, field), field)))\
774 elm = RTEMS_RB_PARENT(elm, field); \
775 elm = RTEMS_RB_PARENT(elm, field); \
781#define RTEMS_RB_GENERATE_PREV(name, type, field, attr) \
784name##_RTEMS_RB_PREV(struct type *elm) \
786 if (RTEMS_RB_LEFT(elm, field)) { \
787 elm = RTEMS_RB_LEFT(elm, field); \
788 while (RTEMS_RB_RIGHT(elm, field)) \
789 elm = RTEMS_RB_RIGHT(elm, field); \
791 if (RTEMS_RB_PARENT(elm, field) && \
792 (elm == RTEMS_RB_RIGHT(RTEMS_RB_PARENT(elm, field), field))) \
793 elm = RTEMS_RB_PARENT(elm, field); \
795 while (RTEMS_RB_PARENT(elm, field) && \
796 (elm == RTEMS_RB_LEFT(RTEMS_RB_PARENT(elm, field), field)))\
797 elm = RTEMS_RB_PARENT(elm, field); \
798 elm = RTEMS_RB_PARENT(elm, field); \
804#define RTEMS_RB_GENERATE_MINMAX(name, type, field, attr) \
806name##_RTEMS_RB_MINMAX(struct name *head, int val) \
808 struct type *tmp = RTEMS_RB_ROOT(head); \
809 struct type *parent = NULL; \
813 tmp = RTEMS_RB_LEFT(tmp, field); \
815 tmp = RTEMS_RB_RIGHT(tmp, field); \
820#define RTEMS_RB_GENERATE_REINSERT(name, type, field, cmp, attr) \
822name##_RTEMS_RB_REINSERT(struct name *head, struct type *elm) \
824 struct type *cmpelm; \
825 if (((cmpelm = RTEMS_RB_PREV(name, head, elm)) != NULL && \
826 cmp(cmpelm, elm) >= 0) || \
827 ((cmpelm = RTEMS_RB_NEXT(name, head, elm)) != NULL && \
828 cmp(elm, cmpelm) >= 0)) { \
830 RTEMS_RB_REMOVE(name, head, elm); \
831 return (RTEMS_RB_INSERT(name, head, elm)); \
836#define RTEMS_RB_NEGINF -1
837#define RTEMS_RB_INF 1
839#define RTEMS_RB_INSERT(name, x, y) name##_RTEMS_RB_INSERT(x, y)
840#define RTEMS_RB_REMOVE(name, x, y) name##_RTEMS_RB_REMOVE(x, y)
841#define RTEMS_RB_FIND(name, x, y) name##_RTEMS_RB_FIND(x, y)
842#define RTEMS_RB_NFIND(name, x, y) name##_RTEMS_RB_NFIND(x, y)
843#define RTEMS_RB_NEXT(name, x, y) name##_RTEMS_RB_NEXT(y)
844#define RTEMS_RB_PREV(name, x, y) name##_RTEMS_RB_PREV(y)
845#define RTEMS_RB_MIN(name, x) name##_RTEMS_RB_MINMAX(x, RTEMS_RB_NEGINF)
846#define RTEMS_RB_MAX(name, x) name##_RTEMS_RB_MINMAX(x, RTEMS_RB_INF)
847#define RTEMS_RB_REINSERT(name, x, y) name##_RTEMS_RB_REINSERT(x, y)
849#define RTEMS_RB_FOREACH(x, name, head) \
850 for ((x) = RTEMS_RB_MIN(name, head); \
852 (x) = name##_RTEMS_RB_NEXT(x))
854#define RTEMS_RB_FOREACH_FROM(x, name, y) \
856 ((x) != NULL) && ((y) = name##_RTEMS_RB_NEXT(x), (x) != NULL); \
859#define RTEMS_RB_FOREACH_SAFE(x, name, head, y) \
860 for ((x) = RTEMS_RB_MIN(name, head); \
861 ((x) != NULL) && ((y) = name##_RTEMS_RB_NEXT(x), (x) != NULL); \
864#define RTEMS_RB_FOREACH_REVERSE(x, name, head) \
865 for ((x) = RTEMS_RB_MAX(name, head); \
867 (x) = name##_RTEMS_RB_PREV(x))
869#define RTEMS_RB_FOREACH_REVERSE_FROM(x, name, y) \
871 ((x) != NULL) && ((y) = name##_RTEMS_RB_PREV(x), (x) != NULL); \
874#define RTEMS_RB_FOREACH_REVERSE_SAFE(x, name, head, y) \
875 for ((x) = RTEMS_RB_MAX(name, head); \
876 ((x) != NULL) && ((y) = name##_RTEMS_RB_PREV(x), (x) != NULL); \