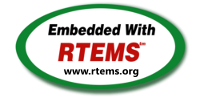 |
RTEMS 6.1-rc5
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
38#ifndef _RTEMS_ZILOG_Z8530_H
39#define _RTEMS_ZILOG_Z8530_H
47#define VOL8( ptr ) ((volatile uint8_t *)(ptr))
49#define Z8x30_STATE0 ( z8530 ) \
51 (garbage) = *(VOL8(z8530)) \
54#define Z8x30_WRITE_CONTROL( z8530, reg, data ) \
55 *(VOL8(z8530)) = (reg); \
56 *(VOL8(z8530)) = (data)
58#define Z8x30_READ_CONTROL( z8530, reg, data ) \
59 *(VOL8(z8530)) = (reg); \
60 (data) = *(VOL8(z8530))
62#define Z8x30_WRITE_DATA( z8530, data ) \
63 *(VOL8(z8530)) = (data);
65#define Z8x30_READ_DATA( z8530, data ) \
66 (data) = *(VOL8(z8530));
71#define RR_0_TX_BUFFER_EMPTY 0x04
72#define RR_0_RX_DATA_AVAILABLE 0x01