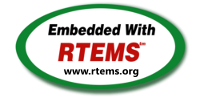 |
RTEMS 6.1-rc5
|
Loading...
Searching...
No Matches
30#ifndef ILI9488_REG_H_INCLUDED
31#define ILI9488_REG_H_INCLUDED
34#define ILI9488_CMD_NOP 0x00
35#define ILI9488_CMD_SOFTWARE_RESET 0x01
36#define ILI9488_CMD_READ_DISP_ID 0x04
37#define ILI9488_CMD_READ_ERROR_DSI 0x05
38#define ILI9488_CMD_READ_DISP_STATUS 0x09
39#define ILI9488_CMD_READ_DISP_POWER_MODE 0x0A
40#define ILI9488_CMD_READ_DISP_MADCTRL 0x0B
41#define ILI9488_CMD_READ_DISP_PIXEL_FORMAT 0x0C
42#define ILI9488_CMD_READ_DISP_IMAGE_MODE 0x0D
43#define ILI9488_CMD_READ_DISP_SIGNAL_MODE 0x0E
44#define ILI9488_CMD_READ_DISP_SELF_DIAGNOSTIC 0x0F
45#define ILI9488_CMD_ENTER_SLEEP_MODE 0x10
46#define ILI9488_CMD_SLEEP_OUT 0x11
47#define ILI9488_CMD_PARTIAL_MODE_ON 0x12
48#define ILI9488_CMD_NORMAL_DISP_MODE_ON 0x13
49#define ILI9488_CMD_DISP_INVERSION_OFF 0x20
50#define ILI9488_CMD_DISP_INVERSION_ON 0x21
51#define ILI9488_CMD_PIXEL_OFF 0x22
52#define ILI9488_CMD_PIXEL_ON 0x23
53#define ILI9488_CMD_DISPLAY_OFF 0x28
54#define ILI9488_CMD_DISPLAY_ON 0x29
55#define ILI9488_CMD_COLUMN_ADDRESS_SET 0x2A
56#define ILI9488_CMD_PAGE_ADDRESS_SET 0x2B
57#define ILI9488_CMD_MEMORY_WRITE 0x2C
58#define ILI9488_CMD_MEMORY_READ 0x2E
59#define ILI9488_CMD_PARTIAL_AREA 0x30
60#define ILI9488_CMD_VERT_SCROLL_DEFINITION 0x33
61#define ILI9488_CMD_TEARING_EFFECT_LINE_OFF 0x34
62#define ILI9488_CMD_TEARING_EFFECT_LINE_ON 0x35
63#define ILI9488_CMD_MEMORY_ACCESS_CONTROL 0x36
64#define ILI9488_CMD_VERT_SCROLL_START_ADDRESS 0x37
65#define ILI9488_CMD_IDLE_MODE_OFF 0x38
66#define ILI9488_CMD_IDLE_MODE_ON 0x39
67#define ILI9488_CMD_COLMOD_PIXEL_FORMAT_SET 0x3A
68#define ILI9488_CMD_WRITE_MEMORY_CONTINUE 0x3C
69#define ILI9488_CMD_READ_MEMORY_CONTINUE 0x3E
70#define ILI9488_CMD_SET_TEAR_SCANLINE 0x44
71#define ILI9488_CMD_GET_SCANLINE 0x45
72#define ILI9488_CMD_WRITE_DISPLAY_BRIGHTNESS 0x51
73#define ILI9488_CMD_READ_DISPLAY_BRIGHTNESS 0x52
74#define ILI9488_CMD_WRITE_CTRL_DISPLAY 0x53
75#define ILI9488_CMD_READ_CTRL_DISPLAY 0x54
76#define ILI9488_CMD_WRITE_CONTENT_ADAPT_BRIGHTNESS 0x55
77#define ILI9488_CMD_READ_CONTENT_ADAPT_BRIGHTNESS 0x56
78#define ILI9488_CMD_WRITE_MIN_CAB_LEVEL 0x5E
79#define ILI9488_CMD_READ_MIN_CAB_LEVEL 0x5F
80#define ILI9488_CMD_READ_ABC_SELF_DIAG_RES 0x68
81#define ILI9488_CMD_READ_ID1 0xDA
82#define ILI9488_CMD_READ_ID2 0xDB
83#define ILI9488_CMD_READ_ID3 0xDC
86#define ILI9488_CMD_INTERFACE_MODE_CONTROL 0xB0
87#define ILI9488_CMD_FRAME_RATE_CONTROL_NORMAL 0xB1
88#define ILI9488_CMD_FRAME_RATE_CONTROL_IDLE_8COLOR 0xB2
89#define ILI9488_CMD_FRAME_RATE_CONTROL_PARTIAL 0xB3
90#define ILI9488_CMD_DISPLAY_INVERSION_CONTROL 0xB4
91#define ILI9488_CMD_BLANKING_PORCH_CONTROL 0xB5
92#define ILI9488_CMD_DISPLAY_FUNCTION_CONTROL 0xB6
93#define ILI9488_CMD_ENTRY_MODE_SET 0xB7
94#define ILI9488_CMD_BACKLIGHT_CONTROL_1 0xB9
95#define ILI9488_CMD_BACKLIGHT_CONTROL_2 0xBA
96#define ILI9488_CMD_HS_LANES_CONTROL 0xBE
97#define ILI9488_CMD_POWER_CONTROL_1 0xC0
98#define ILI9488_CMD_POWER_CONTROL_2 0xC1
99#define ILI9488_CMD_POWER_CONTROL_NORMAL_3 0xC2
100#define ILI9488_CMD_POWER_CONTROL_IDEL_4 0xC3
101#define ILI9488_CMD_POWER_CONTROL_PARTIAL_5 0xC4
102#define ILI9488_CMD_VCOM_CONTROL_1 0xC5
103#define ILI9488_CMD_CABC_CONTROL_1 0xC6
104#define ILI9488_CMD_CABC_CONTROL_2 0xC8
105#define ILI9488_CMD_CABC_CONTROL_3 0xC9
106#define ILI9488_CMD_CABC_CONTROL_4 0xCA
107#define ILI9488_CMD_CABC_CONTROL_5 0xCB
108#define ILI9488_CMD_CABC_CONTROL_6 0xCC
109#define ILI9488_CMD_CABC_CONTROL_7 0xCD
110#define ILI9488_CMD_CABC_CONTROL_8 0xCE
111#define ILI9488_CMD_CABC_CONTROL_9 0xCF
112#define ILI9488_CMD_NVMEM_WRITE 0xD0
113#define ILI9488_CMD_NVMEM_PROTECTION_KEY 0xD1
114#define ILI9488_CMD_NVMEM_STATUS_READ 0xD2
115#define ILI9488_CMD_READ_ID4 0xD3
116#define ILI9488_CMD_ADJUST_CONTROL_1 0xD7
117#define ILI9488_CMD_READ_ID_VERSION 0xD8
118#define ILI9488_CMD_POSITIVE_GAMMA_CORRECTION 0xE0
119#define ILI9488_CMD_NEGATIVE_GAMMA_CORRECTION 0xE1
120#define ILI9488_CMD_DIGITAL_GAMMA_CONTROL_1 0xE2
121#define ILI9488_CMD_DIGITAL_GAMMA_CONTROL_2 0xE3
122#define ILI9488_CMD_SET_IMAGE_FUNCTION 0xE9
123#define ILI9488_CMD_ADJUST_CONTROL_2 0xF2
124#define ILI9488_CMD_ADJUST_CONTROL_3 0xF7
125#define ILI9488_CMD_ADJUST_CONTROL_4 0xF8
126#define ILI9488_CMD_ADJUST_CONTROL_5 0xF9
127#define ILI9488_CMD_SPI_READ_SETTINGS 0xFB
128#define ILI9488_CMD_ADJUST_CONTROL_6 0xFC
129#define ILI9488_CMD_ADJUST_CONTROL_7 0xFF