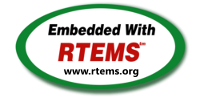 |
RTEMS 6.1-rc5
|
Loading...
Searching...
No Matches
1#ifndef __TASK_API_BESTCOMM_CNTRL_H
2#define __TASK_API_BESTCOMM_CNTRL_H 1
33#define SDMA_INT_BIT_DBG 31
34#define SDMA_INT_BIT_TEA 28
35#define SDMA_INT_BIT_TEA_TASK 24
36#define SDMA_INT_BIT_IMPL 0x9000FFFF
38#define SDMA_PTDCTRL_BIT_TEA 14
40#define SDMA_TCR_BIT_AUTO 15
41#define SDMA_TCR_BIT_HOLD 5
43#define SDMA_STAT_BIT_ALARM 17
44#define SDMA_FIFO_ALARM_MASK 0x0020000
46#define SDMA_DRD_BIT_TFD 27
47#define SDMA_DRD_BIT_INT 26
48#define SDMA_DRD_BIT_INIT 21
49#define SDMA_DRD_MASK_FLAGS 0x0C000000
50#define SDMA_DRD_MASK_LENGTH 0x03FFFFFF
51#define SDMA_BD_BIT_READY 30
53 #define SDMA_BD_MASK_READY constant(1<<SDMA_BD_BIT_READY)
55 #define SDMA_BD_MASK_READY (1<<SDMA_BD_BIT_READY)
57#define SDMA_BD_MASK_SIGN 0x7FFFFFFF
59#define SDMA_PRAGMA_BIT_RSV 7
60#define SDMA_PRAGMA_BIT_PRECISE_INC 6
61#define SDMA_PRAGMA_BIT_RST_ERROR_NO 5
62#define SDMA_PRAGMA_BIT_PACK 4
63#define SDMA_PRAGMA_BIT_INTEGER 3
64#define SDMA_PRAGMA_BIT_SPECREAD 2
65#define SDMA_PRAGMA_BIT_CW 1
66#define SDMA_PRAGMA_BIT_RL 0
68#define SDMA_TASK_ENTRY_BYTES 32
69#define SDMA_TASK_GROUP_NUM 16
70#define SDMA_TASK_GROUP_BYTES (SDMA_TASK_ENTRY_BYTES*SDMA_TASK_GROUP_NUM)
76#define SDMA_TASKNUM_EXT(OldTaskNum) (OldTaskNum%16)
78#define SDMA_TASKBAR_CHANGE(sdma, OldTaskNum) { \
79 sdma->taskBar += (((int)(OldTaskNum/SDMA_TASK_GROUP_NUM))*SDMA_TASK_GROUP_BYTES); \
82#define SDMA_TASKBAR_RESTORE(sdma, OldTaskNum) { \
83 sdma->taskBar -= (((int)(OldTaskNum/SDMA_TASK_GROUP_NUM))*SDMA_TASK_GROUP_BYTES); \
90#define SDMA_TASK_CFG(RegAddr, TaskNum, AutoStart, AutoStartNum) { \
91 *(((volatile uint16 *)RegAddr)+TaskNum) = (uint16)(0x0000 | \
92 ((AutoStart!=0)<<7) | \
93 (AutoStartNum&0xF) ); \
96#define SDMA_TASK_AUTO_START(RegAddr, TaskNum, AutoStart, AutoStartNum) { \
97 *(((volatile uint16 *)RegAddr)+TaskNum) = (uint16)((*(((volatile uint16 *)RegAddr)+TaskNum) & \
98 (uint16) 0xff30) | ((uint16)(0x0000 | \
99 ((AutoStart!=0)<<7) | \
100 (AutoStartNum&0xF)) )); \
103#define SDMA_TASK_ENABLE(RegAddr, TaskNum) { \
104 *(((volatile uint16 *)RegAddr)+TaskNum) |= (uint16)0x8000; \
107#define SDMA_TASK_DISABLE(RegAddr, TaskNum) { \
108 *(((volatile uint16 *)RegAddr)+TaskNum) &= ~(uint16)0x8000; \
111#define SDMA_TASK_STATUS(RegAddr, TaskNum) \
112 *(((volatile uint16 *)RegAddr)+TaskNum)
118#define SDMA_INT_ENABLE(RegAddr, Bit) \
120 rtems_interrupt_level level; \
121 rtems_interrupt_disable(level); \
122 *((volatile uint32 *) RegAddr) &= ~((uint32) (1 << Bit)); \
123 rtems_interrupt_enable(level); \
126#define SDMA_INT_DISABLE(RegAddr, Bit) \
128 rtems_interrupt_level level; \
129 rtems_interrupt_disable(level); \
130 *((volatile uint32 *) (RegAddr)) |= ((uint32)(1 << Bit)); \
131 rtems_interrupt_enable(level); \
134#define SDMA_INT_SOURCE(RegPend, RegMask) \
135 (*((volatile uint32 *)(RegPend)) & (~*((volatile uint32 *)(RegMask))) & (uint32)SDMA_INT_BIT_IMPL)
137#define SDMA_INT_PENDING(RegPend, RegMask) \
138 (*((volatile uint32 *)(RegPend)) & (~*((volatile uint32 *)(RegMask))))
140#define SDMA_INT_TEST(IntSource, Bit) \
141 (((uint32)IntSource) & ((uint32)(1<<Bit)))
149#define SDMA_CLEAR_IEVENT(RegAddr, Bit) { \
150 *((volatile uint32 *)RegAddr) = ((uint32)(1<<Bit)); \
153#define SDMA_GET_PENDINGBIT(sdma, Bit) \
154 (sdma->IntPend & (uint32)(1<<Bit))
156#define SDMA_GET_MASKBIT(sdma, Bit) \
157 (sdma->IntMask & (uint32)(1<<Bit))
168#define SDMA_TEA_ENABLE(sdma) { \
169 SDMA_INT_ENABLE(sdma, SDMA_INT_BIT_TEA); \
170 sdma->PtdCntrl &= ~((uint32)(1<<SDMA_PTDCTRL_BIT_TEA)); \
174#define SDMA_TEA_DISABLE(sdma) { \
175 SDMA_INT_DISABLE(sdma, SDMA_INT_BIT_TEA); \
176 sdma->PtdCntrl |= ((uint32)(1<<SDMA_PTDCTRL_BIT_TEA)); \
180#define SDMA_TEA_CLEAR(sdma) { \
181 sdma->IntPend = ((uint32)(0x1F<<SDMA_INT_BIT_TEA_TASK)); \
185#define SDMA_TEA_SOURCE(RegPend) \
186 (uint32)(((*(volatile uint32 *)RegPend)>>SDMA_INT_BIT_TEA_TASK) & 0xF)
194#define SDMA_DBG_ENABLE(sdma) { \
195 SDMA_INT_ENABLE(sdma, SDMA_INT_BIT_DBG); \
198#define SDMA_DBG_DISABLE(sdma) { \
199 SDMA_INT_DISABLE(sdma, SDMA_INT_BIT_DBG); \
203#define SDMA_DBG_CLEAR(sdma) { \
204 SDMA_CLEAR_IEVENT(sdma, SDMA_INT_BIT_DBG); \
207#define SDMA_DBG_MDE(dst, sdma, addr) { \
208 sdma->MDEDebug = addr; \
209 dst = sdma->MDEDebug; \
212#define SDMA_DBG_ADS(dst, sdma, addr) { \
213 sdma->ADSDebug = addr; \
214 dst = sdma->ADSDebug; \
217#define SDMA_DBG_PTD(dst, sdma, addr) { \
218 sdma->PTDDebug = addr; \
219 dst = sdma->PTDDebug; \
231#define SDMA_SET_SIZE(RegAddr, TaskNum, SrcSize, DstSize) \
232 *(((volatile uint8 *)RegAddr)+((uint32)(TaskNum/2))) = \
233 (uint8)((*(((volatile uint8 *)RegAddr)+((uint32)(TaskNum/2))) & \
234 ((TaskNum%2) ? 0xf0 : 0x0f)) | \
235 ((uint8)(((SrcSize & 0x3)<<2) | \
236 ( DstSize & 0x3 ) ) <<(4*((int)(1-(TaskNum%2))))));
240#define SDMA_SET_INIT(RegAddr, TaskNum, Initiator) \
242 *(((volatile uint16 *)RegAddr)+TaskNum) &= (uint16)0xE0FF; \
243 *(((volatile uint16 *)RegAddr)+TaskNum) |= (((0x01F & Initiator)<<8) | \
244 (0<<SDMA_TCR_BIT_HOLD)); \
248#define SDMA_INIT_CHANGE(task, oldInitiator, newInitiator) { \
250 for (i=0; i<task->NumDRD; i++) { \
251 if (SDMA_INIT_READ(task->DRD[i]) == (uint32)oldInitiator) { \
252 SDMA_INIT_WRITE(task->DRD[i],newInitiator); \
258#define SDMA_SET_INITIATOR_PRIORITY(sdma, initiator, priority) \
259 *(((volatile uint8 *)&sdma->IPR0)+initiator) = priority;
263#define SDMA_INIT_READ(PtrDRD) \
264 (((*(volatile uint32 *)PtrDRD)>>SDMA_DRD_BIT_INIT) & (uint32)0x1F)
267#define SDMA_INIT_WRITE(PtrDRD, Initiator) { \
268 *(volatile uint32 *)PtrDRD = ((*(volatile uint32 *)PtrDRD) & 0xFC1FFFFF) | \
269 (Initiator<<SDMA_DRD_BIT_INIT); \
273#define SDMA_INIT_CHANGE(task, oldInitiator, newInitiator) { \
275 for (i=0; i<task->NumDRD; i++) { \
276 if (SDMA_INIT_READ(task->DRD[i]) == (uint32)oldInitiator) { \
277 SDMA_INIT_WRITE(task->DRD[i],newInitiator); \