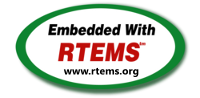 |
RTEMS 6.1-rc4
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
38#define TX4925_REG_BASE 0xFF1F0000
44#define TX4925_CFG_CCFG 0xE000
45#define TX4925_CFG_REVID 0xE004
46#define TX4925_CFG_PCFG 0xE008
47#define TX4925_CFG_TOEA 0xE00C
48#define TX4925_CFG_PDNCTR 0xE010
49#define TX4925_CFG_GARBP 0xE018
50#define TX4925_CFG_TOCNT 0xE020
51#define TX4925_CFG_DRQCTR 0xE024
52#define TX4925_CFG_CLKCTR 0xE028
53#define TX4925_CFG_GARBC 0xE02C
54#define TX4925_CFG_RAMP 0xE030
57#define SELCHI 0x00100000
58#define SELTMR0 0x00000200
65#define TX4925_TIMER0_BASE 0xF000
66#define TX4925_TIMER1_BASE 0xF100
67#define TX4925_TIMER2_BASE 0xF200
69#define TX4925_TIMER_TCR 0x00
70#define TX4925_TIMER_TISR 0x04
71#define TX4925_TIMER_CPRA 0x08
72#define TX4925_TIMER_CPRB 0x0C
73#define TX4925_TIMER_ITMR 0x10
74#define TX4925_TIMER_CCDR 0x20
75#define TX4925_TIMER_PGMR 0x30
76#define TX4925_TIMER_WTMR 0x40
77#define TX4925_TIMER_TRR 0xF0
80#define TIMER_CLEAR_ENABLE_MASK 0x1
81#define TIMER_INT_ENABLE_MASK 0x8000
98#define TX4925_IRQCTL_DEN 0xF600
99#define TX4925_IRQCTL_DM0 0xF604
100#define TX4925_IRQCTL_DM1 0xF608
101#define TX4925_IRQCTL_LVL0 0xF610
102#define TX4925_IRQCTL_LVL1 0xF614
103#define TX4925_IRQCTL_LVL2 0xF618
104#define TX4925_IRQCTL_LVL3 0xF61C
105#define TX4925_IRQCTL_LVL4 0xF620
106#define TX4925_IRQCTL_LVL5 0xF624
107#define TX4925_IRQCTL_LVL6 0xF628
108#define TX4925_IRQCTL_LVL7 0xF62C
109#define TX4925_IRQCTL_MSK 0xF640
110#define TX4925_IRQCTL_EDC 0xF660
111#define TX4925_IRQCTL_PND 0xF680
112#define TX4925_IRQCTL_CS 0xF6A0
113#define TX4925_IRQCTL_FLAG0 0xF510
114#define TX4925_IRQCTL_FLAG1 0xF514
115#define TX4925_IRQCTL_POL 0xF518
116#define TX4925_IRQCTL_RCNT 0xF51C
117#define TX4925_IRQCTL_MASKINT 0xF520
118#define TX4925_IRQCTL_MASKEXT 0xF524
120#define TX4925_REG_READ( _base, _register ) \
121 *((volatile uint32_t *)((_base) + (_register)))
123#define TX4925_REG_WRITE( _base, _register, _value ) \
124 *((volatile uint32_t *)((_base) + (_register))) = (_value)