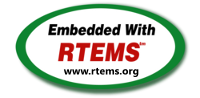 |
RTEMS 6.1-rc4
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
44#ifndef _SYS_PRIORITY_H_
45#define _SYS_PRIORITY_H_
57#define PRI_TIMESHARE 3
77#define PRI_MIN_ITHD (PRI_MIN)
78#define PRI_MAX_ITHD (PRI_MIN_REALTIME - 1)
80#define PI_REALTIME (PRI_MIN_ITHD + 0)
81#define PI_AV (PRI_MIN_ITHD + 4)
82#define PI_NET (PRI_MIN_ITHD + 8)
83#define PI_DISK (PRI_MIN_ITHD + 12)
84#define PI_TTY (PRI_MIN_ITHD + 16)
85#define PI_DULL (PRI_MIN_ITHD + 20)
86#define PI_SOFT (PRI_MIN_ITHD + 24)
87#define PI_SWI(x) (PI_SOFT + (x) * RQ_PPQ)
89#define PRI_MIN_REALTIME (48)
90#define PRI_MAX_REALTIME (PRI_MIN_KERN - 1)
92#define PRI_MIN_KERN (80)
93#define PRI_MAX_KERN (PRI_MIN_TIMESHARE - 1)
95#define PSWP (PRI_MIN_KERN + 0)
96#define PVM (PRI_MIN_KERN + 4)
97#define PINOD (PRI_MIN_KERN + 8)
98#define PRIBIO (PRI_MIN_KERN + 12)
99#define PVFS (PRI_MIN_KERN + 16)
100#define PZERO (PRI_MIN_KERN + 20)
101#define PSOCK (PRI_MIN_KERN + 24)
102#define PWAIT (PRI_MIN_KERN + 28)
103#define PLOCK (PRI_MIN_KERN + 32)
104#define PPAUSE (PRI_MIN_KERN + 36)
106#define PRI_MIN_TIMESHARE (120)
107#define PRI_MAX_TIMESHARE (PRI_MIN_IDLE - 1)
109#define PUSER (PRI_MIN_TIMESHARE)
111#define PRI_MIN_IDLE (224)
112#define PRI_MAX_IDLE (PRI_MAX)
117#define PRI_UNCHANGED -1