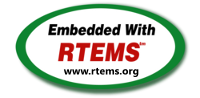 |
RTEMS 6.1-rc4
|
Loading...
Searching...
No Matches
38extern void grpwm_register_drv (
void);
40#define GRPWM_IOCTL_GET_CAP 1
41#define GRPWM_IOCTL_SET_CONFIG 2
42#define GRPWM_IOCTL_SET_SCALER 3
43#define GRPWM_IOCTL_UPDATE 4
44#define GRPWM_IOCTL_IRQ 5
63 unsigned char dbscaler;
64 unsigned char scaler_index;
67 unsigned char irqscaler;
69 void (*isr)(
int channel,
void *arg);
73 unsigned int wave_synccfg;
74 unsigned int wave_sync;
75 unsigned int *wave_data;
76 unsigned int wave_data_length;
79#define GRPWM_CONFIG_OPTION_FLIP 0x04000000
80#define GRPWM_CONFIG_OPTION_DEAD_BAND 0x00200000
81#define GRPWM_CONFIG_OPTION_SYMMETRIC 0x00000040
82#define GRPWM_CONFIG_OPTION_ASYMMERTIC 0
83#define GRPWM_CONFIG_OPTION_DUAL 0x00000020
84#define GRPWM_CONFIG_OPTION_PAIR 0x00000004
85#define GRPWM_CONFIG_OPTION_SINGLE 0x00000000
86#define GRPWM_CONFIG_OPTION_POLARITY_HIGH 0x00000002
87#define GRPWM_CONFIG_OPTION_POLARITY_LOW 0x00000000
89#define GRPWM_CONFIG_OPTION_MASK ( \
90 GRPWM_CONFIG_OPTION_DEAD_BAND | GRPWM_CONFIG_OPTION_SYMMETRIC | \
91 GRPWM_CONFIG_OPTION_DUAL | GRPWM_CONFIG_OPTION_PAIR | \
92 GRPWM_CONFIG_OPTION_POLARITY_HIGH \
98 unsigned int index_mask;
99 unsigned int values[8];
104#define GRPWM_UPDATE_OPTION_ENABLE 0x01
105#define GRPWM_UPDATE_OPTION_DISABLE 0x02
106#define GRPWM_UPDATE_OPTION_PERIOD 0x04
107#define GRPWM_UPDATE_OPTION_COMP 0x08
108#define GRPWM_UPDATE_OPTION_DBCOMP 0x10
109#define GRPWM_UPDATE_OPTION_FIX 0x20
112#define GRPWM_UPDATE_FIX_ENABLE 1
113#define GRPWM_UPDATE_FIX_DISABLE 0
114#define GRPWM_UPDATE_FIX_0_LOW 0
115#define GRPWM_UPDATE_FIX_0_HIGH 2
116#define GRPWM_UPDATE_FIX_1_LOW 0
117#define GRPWM_UPDATE_FIX_1_HIGH 4
120 unsigned int options;
122 unsigned int compare;
129 unsigned char chanmask;
135#define GRPWM_IRQ_DISABLE 0
136#define GRPWM_IRQ_PERIOD 1
137#define GRPWM_IRQ_COMPARE 3
138#define GRPWM_IRQ_CLEAR 0x10
140#define GRPWM_IRQ_CHAN 0x100