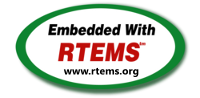 |
RTEMS 6.1-rc4
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
31#define FBIOGET_VSCREENINFO 0x4600
32#define FBIOPUT_VSCREENINFO 0x4601
33#define FBIOGET_FSCREENINFO 0x4602
34#define FBIOGETCMAP 0x4604
35#define FBIOPUTCMAP 0x4605
36#define FB_EXEC_FUNCTION 0x4606
37#define FBIOSWAPBUFFERS 0x4607
38#define FBIOSETBUFFERMODE 0x4608
39#define FBIOSETVIDEOMODE 0x4609
41#define FB_SINGLE_BUFFERED 0
42#define FB_TRIPLE_BUFFERED 1
44#define FB_TYPE_PACKED_PIXELS 0
45#define FB_TYPE_PLANES 1
46#define FB_TYPE_INTERLEAVED_PLANES 2
48#define FB_TYPE_VGA_PLANES 4
49#define FB_TYPE_VIRTUAL_BUFFER 5
52#define FB_VISUAL_MONO01 0
53#define FB_VISUAL_MONO10 1
54#define FB_VISUAL_TRUECOLOR 2
55#define FB_VISUAL_PSEUDOCOLOR 3
56#define FB_VISUAL_DIRECTCOLOR 4
57#define FB_VISUAL_STATIC_PSEUDOCOLOR 5
59#define FB_ACCEL_NONE 0
71 uint32_t bits_per_pixel;
79 volatile char *smem_start;