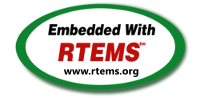 |
RTEMS 6.1-rc2
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
40#ifndef _RTEMS_SCORE_V850_H
41#define _RTEMS_SCORE_V850_H
59#if defined(rtems_multilib)
64#define CPU_MODEL_NAME "rtems_multilib"
66#define V850_HAS_BYTE_SWAP_INSTRUCTION 0
68#elif defined(__v850e2v3__)
69#define CPU_MODEL_NAME "v850e2v3"
71#define V850_HAS_BYTE_SWAP_INSTRUCTION 1
73#elif defined(__v850e2__)
74#define CPU_MODEL_NAME "v850e2"
76#define V850_HAS_BYTE_SWAP_INSTRUCTION 1
78#elif defined(__v850es__)
79#define CPU_MODEL_NAME "v850es"
81#define V850_HAS_BYTE_SWAP_INSTRUCTION 1
83#elif defined(__v850e1__)
84#define CPU_MODEL_NAME "v850e1"
86#define V850_HAS_BYTE_SWAP_INSTRUCTION 1
88#elif defined(__v850e__)
89#define CPU_MODEL_NAME "v850e"
91#define V850_HAS_BYTE_SWAP_INSTRUCTION 1
94#define CPU_MODEL_NAME "v850"
96#define V850_HAS_BYTE_SWAP_INSTRUCTION 0
103#define CPU_NAME "v850 CPU"
108#define v850_set_psw( _psw ) \
109 __asm__ __volatile__( "ldsr %0, psw" : : "r" (_psw) )
114#define v850_get_psw( _psw ) \
115 __asm__ __volatile__( "stsr psw, %0" : "=&r" (_psw) )
120#define V850_PSW_ZERO_MASK 0x01
121#define V850_PSW_IS_ZERO 0x01
122#define V850_PSW_IS_NOT 0x00
124#define V850_PSW_SIGN_MASK 0x02
125#define V850_PSW_SIGN_IS_NEGATIVE 0x02
126#define V850_PSW_SIGN_IS_ZERO_OR_POSITIVE 0x00
128#define V850_PSW_OVERFLOW_MASK 0x02
129#define V850_PSW_OVERFLOW_OCCURRED 0x02
130#define V850_PSW_OVERFLOW_DID_NOT_OCCUR 0x00
132#define V850_PSW_CARRY_OR_BORROW_MASK 0x04
133#define V850_PSW_CARRY_OR_BORROW_OCCURRED 0x04
134#define V850_PSW_CARRY_OR_BORROW_DID_NOT_OCCUR 0x00
136#define V850_PSW_SATURATION_MASK 0x10
137#define V850_PSW_SATURATION_OCCURRED 0x10
138#define V850_PSW_SATURATION_DID_NOT_OCCUR 0x00
140#define V850_PSW_INTERRUPT_DISABLE_MASK 0x20
141#define V850_PSW_INTERRUPT_DISABLE 0x20
142#define V850_PSW_INTERRUPT_ENABLE 0x00
144#define V850_PSW_EXCEPTION_IN_PROCESS_MASK 0x40
145#define V850_PSW_EXCEPTION_IN_PROCESS 0x40
146#define V850_PSW_EXCEPTION_NOT_IN_PROCESS 0x00
148#define V850_PSW_NMI_IN_PROCESS_MASK 0x80
149#define V850_PSW_NMI_IN_PROCESS 0x80
150#define V850_PSW_NMI_NOT_IN_PROCESS 0x00