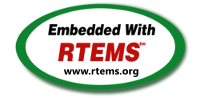 |
RTEMS 6.1-rc2
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
58#if defined(_POSIX_ASYNCHRONOUS_IO)
75#define AIO_NOTCANCELED 1
107 volatile void *aio_buf;
110 struct sigevent aio_sigevent;
114 ssize_t return_value;
139 struct aiocb *__restrict
const list[__restrict],
141 struct sigevent *__restrict sig
149 const struct aiocb *aiocbp
158 const struct aiocb *aiocbp
185 const struct aiocb *
const list[],
187 const struct timespec *timeout
190#if defined(_POSIX_SYNCHRONIZED_IO)