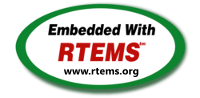 |
RTEMS
5.1
|
39 #ifndef LIBBSP_ARM_TMS570_LIN 40 #define LIBBSP_ARM_TMS570_LIN 77 uint8_t reserved1 [16];
84 #define TMS570_LIN_GCR0_Reserved(val) BSP_FLD32(val,1, 31) 85 #define TMS570_LIN_GCR0_Reserved_GET(reg) BSP_FLD32GET(reg,1, 31) 86 #define TMS570_LIN_GCR0_Reserved_SET(reg,val) BSP_FLD32SET(reg, val,1, 31) 89 #define TMS570_LIN_GCR0_RESET BSP_BIT32(0) 94 #define TMS570_LIN_GCR1_TXENA BSP_BIT32(25) 97 #define TMS570_LIN_GCR1_RXENA BSP_BIT32(24) 100 #define TMS570_LIN_GCR1_CONT BSP_BIT32(17) 103 #define TMS570_LIN_GCR1_LOOP_BACK BSP_BIT32(16) 106 #define TMS570_LIN_GCR1_POWERDOWN BSP_BIT32(9) 109 #define TMS570_LIN_GCR1_SLEEP BSP_BIT32(8) 112 #define TMS570_LIN_GCR1_SWnRST BSP_BIT32(7) 115 #define TMS570_LIN_GCR1_CLOCK BSP_BIT32(5) 118 #define TMS570_LIN_GCR1_STOP BSP_BIT32(4) 121 #define TMS570_LIN_GCR1_PARITY BSP_BIT32(3) 124 #define TMS570_LIN_GCR1_PARITY_ENA BSP_BIT32(2) 127 #define TMS570_LIN_GCR1_TIMING_MODE BSP_BIT32(1) 130 #define TMS570_LIN_GCR1_COMM_MODE BSP_BIT32(0) 135 #define TMS570_LIN_GCR2_CC BSP_BIT32(17) 138 #define TMS570_LIN_GCR2_SC BSP_BIT32(16) 141 #define TMS570_LIN_GCR2_GEN_WU BSP_BIT32(8) 144 #define TMS570_LIN_GCR2_POWERDOWN BSP_BIT32(0) 149 #define TMS570_LIN_SETINT_SET_FE_INT BSP_BIT32(26) 152 #define TMS570_LIN_SETINT_SET_OE_INT BSP_BIT32(25) 155 #define TMS570_LIN_SETINT_SET_PE_INT BSP_BIT32(24) 158 #define TMS570_LIN_SETINT_SET_RX_DMA_ALL BSP_BIT32(18) 161 #define TMS570_LIN_SETINT_SET_RX_DMA BSP_BIT32(17) 164 #define TMS570_LIN_SETINT_SET_TX_DMA BSP_BIT32(16) 167 #define TMS570_LIN_SETINT_SET_RX_INT BSP_BIT32(9) 170 #define TMS570_LIN_SETINT_SET_TX_INT BSP_BIT32(8) 173 #define TMS570_LIN_SETINT_SET_WAKEUP_INT BSP_BIT32(1) 176 #define TMS570_LIN_SETINT_SET_BRKDT_INT BSP_BIT32(0) 181 #define TMS570_LIN_CLEARINT_CLR_FE_INT BSP_BIT32(26) 184 #define TMS570_LIN_CLEARINT_CLR_CE_INT BSP_BIT32(25) 187 #define TMS570_LIN_CLEARINT_CLR_PE_INT BSP_BIT32(24) 190 #define TMS570_LIN_CLEARINT_CLR_RX_DMA_ALL BSP_BIT32(18) 193 #define TMS570_LIN_CLEARINT_CLR_RX_DMA BSP_BIT32(17) 196 #define TMS570_LIN_CLEARINT_CLR_TX_DMA BSP_BIT32(16) 199 #define TMS570_LIN_CLEARINT_CLR_RX_INT BSP_BIT32(9) 202 #define TMS570_LIN_CLEARINT_CLR_TX_INT BSP_BIT32(8) 205 #define TMS570_LIN_CLEARINT_CLR_WAKEUP_INT BSP_BIT32(1) 208 #define TMS570_LIN_CLEARINT_CLR_BRKDT_INT BSP_BIT32(0) 213 #define TMS570_LIN_SETINTLVL_SET_FE_INT_LVL BSP_BIT32(26) 216 #define TMS570_LIN_SETINTLVL_SET_CE_INT_LVL BSP_BIT32(25) 219 #define TMS570_LIN_SETINTLVL_SET_PE_INT_LVL BSP_BIT32(24) 222 #define TMS570_LIN_SETINTLVL_SET_RX_DMA_ALL_LVL BSP_BIT32(18) 225 #define TMS570_LIN_SETINTLVL_SET_RX_INT_LVL BSP_BIT32(9) 228 #define TMS570_LIN_SETINTLVL_SET_TX_INT_LVL BSP_BIT32(8) 231 #define TMS570_LIN_SETINTLVL_SET_WAKEUP_INT_LVL BSP_BIT32(1) 234 #define TMS570_LIN_SETINTLVL_SET_BRKDT_INT_LVL BSP_BIT32(0) 239 #define TMS570_LIN_CLEARINTLVL_CLR_FE_INT_LVL BSP_BIT32(26) 242 #define TMS570_LIN_CLEARINTLVL_CLR_CE_INT_LVL BSP_BIT32(25) 245 #define TMS570_LIN_CLEARINTLVL_CLR_CE_INT_LVL BSP_BIT32(25) 248 #define TMS570_LIN_CLEARINTLVL_CLR_PE_INT_LVL BSP_BIT32(24) 251 #define TMS570_LIN_CLEARINTLVL_CLR_RX_DMA_ALL_LVL BSP_BIT32(18) 254 #define TMS570_LIN_CLEARINTLVL_CLR_RX_INT_LVL BSP_BIT32(9) 257 #define TMS570_LIN_CLEARINTLVL_8 BSP_BIT32(8) 260 #define TMS570_LIN_CLEARINTLVL_CLR_WAKEUP_INT_LVL BSP_BIT32(1) 263 #define TMS570_LIN_CLEARINTLVL_CLR_BRKDT_INT_LVL BSP_BIT32(0) 268 #define TMS570_LIN_FLR_FE BSP_BIT32(26) 271 #define TMS570_LIN_FLR_OE BSP_BIT32(25) 274 #define TMS570_LIN_FLR_PE BSP_BIT32(24) 277 #define TMS570_LIN_FLR_RXWAKE BSP_BIT32(12) 280 #define TMS570_LIN_FLR_TX_EMPTY BSP_BIT32(11) 283 #define TMS570_LIN_FLR_TXWAKE BSP_BIT32(10) 286 #define TMS570_LIN_FLR_RXRDY BSP_BIT32(9) 289 #define TMS570_LIN_FLR_TXRDY BSP_BIT32(8) 292 #define TMS570_LIN_FLR_BUSY BSP_BIT32(3) 295 #define TMS570_LIN_FLR_IDLE BSP_BIT32(2) 298 #define TMS570_LIN_FLR_WAKEUP BSP_BIT32(1) 301 #define TMS570_LIN_FLR_BRKDT BSP_BIT32(0) 306 #define TMS570_LIN_INTVECT0_INVECT0(val) BSP_FLD32(val,0, 3) 307 #define TMS570_LIN_INTVECT0_INVECT0_GET(reg) BSP_FLD32GET(reg,0, 3) 308 #define TMS570_LIN_INTVECT0_INVECT0_SET(reg,val) BSP_FLD32SET(reg, val,0, 3) 313 #define TMS570_LIN_INTVECT1_INVECT1(val) BSP_FLD32(val,0, 3) 314 #define TMS570_LIN_INTVECT1_INVECT1_GET(reg) BSP_FLD32GET(reg,0, 3) 315 #define TMS570_LIN_INTVECT1_INVECT1_SET(reg,val) BSP_FLD32SET(reg, val,0, 3) 320 #define TMS570_LIN_FORMAT_CHAR(val) BSP_FLD32(val,0, 2) 321 #define TMS570_LIN_FORMAT_CHAR_GET(reg) BSP_FLD32GET(reg,0, 2) 322 #define TMS570_LIN_FORMAT_CHAR_SET(reg,val) BSP_FLD32SET(reg, val,0, 2) 327 #define TMS570_LIN_BRS_BAUD(val) BSP_FLD32(val,0, 23) 328 #define TMS570_LIN_BRS_BAUD_GET(reg) BSP_FLD32GET(reg,0, 23) 329 #define TMS570_LIN_BRS_BAUD_SET(reg,val) BSP_FLD32SET(reg, val,0, 23) 334 #define TMS570_LIN_ED_ED(val) BSP_FLD32(val,0, 7) 335 #define TMS570_LIN_ED_ED_GET(reg) BSP_FLD32GET(reg,0, 7) 336 #define TMS570_LIN_ED_ED_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 341 #define TMS570_LIN_RD_RD(val) BSP_FLD32(val,0, 7) 342 #define TMS570_LIN_RD_RD_GET(reg) BSP_FLD32GET(reg,0, 7) 343 #define TMS570_LIN_RD_RD_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 348 #define TMS570_LIN_TD_TD(val) BSP_FLD32(val,0, 7) 349 #define TMS570_LIN_TD_TD_GET(reg) BSP_FLD32GET(reg,0, 7) 350 #define TMS570_LIN_TD_TD_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 355 #define TMS570_LIN_PIO0_TX_FUNC BSP_BIT32(2) 358 #define TMS570_LIN_PIO0_RX_FUNC BSP_BIT32(1) 363 #define TMS570_LIN_PIO1_TX_DIR BSP_BIT32(2) 366 #define TMS570_LIN_PIO1_RX_DIR BSP_BIT32(1) 371 #define TMS570_LIN_PIO2_TX_IN BSP_BIT32(2) 374 #define TMS570_LIN_PIO2_RX_IN BSP_BIT32(1) 379 #define TMS570_LIN_PIO3_TX_OUT BSP_BIT32(2) 382 #define TMS570_LIN_PIO3_RX_OUT BSP_BIT32(1) 387 #define TMS570_LIN_PIO4_TX_SET BSP_BIT32(2) 390 #define TMS570_LIN_PIO4_RX_SET BSP_BIT32(1) 395 #define TMS570_LIN_PIO5_TX_CLR BSP_BIT32(2) 398 #define TMS570_LIN_PIO5_RX_CLR BSP_BIT32(1) 403 #define TMS570_LIN_PIO6_TX_PDR BSP_BIT32(2) 406 #define TMS570_LIN_PIO6_RX_PDR BSP_BIT32(1) 411 #define TMS570_LIN_PIO7_TX_PD BSP_BIT32(2) 414 #define TMS570_LIN_PIO7_RX_PD BSP_BIT32(1) 419 #define TMS570_LIN_PIO8_TX_PSL BSP_BIT32(2) 422 #define TMS570_LIN_PIO8_RX_PSL BSP_BIT32(1) 427 #define TMS570_LIN_COMP_SDEL(val) BSP_FLD32(val,8, 9) 428 #define TMS570_LIN_COMP_SDEL_GET(reg) BSP_FLD32GET(reg,8, 9) 429 #define TMS570_LIN_COMP_SDEL_SET(reg,val) BSP_FLD32SET(reg, val,8, 9) 432 #define TMS570_LIN_COMP_SBREAK(val) BSP_FLD32(val,0, 2) 433 #define TMS570_LIN_COMP_SBREAK_GET(reg) BSP_FLD32GET(reg,0, 2) 434 #define TMS570_LIN_COMP_SBREAK_SET(reg,val) BSP_FLD32SET(reg, val,0, 2) 439 #define TMS570_LIN_RD0_RD0(val) BSP_FLD32(val,24, 31) 440 #define TMS570_LIN_RD0_RD0_GET(reg) BSP_FLD32GET(reg,24, 31) 441 #define TMS570_LIN_RD0_RD0_SET(reg,val) BSP_FLD32SET(reg, val,24, 31) 444 #define TMS570_LIN_RD0_RD1(val) BSP_FLD32(val,16, 23) 445 #define TMS570_LIN_RD0_RD1_GET(reg) BSP_FLD32GET(reg,16, 23) 446 #define TMS570_LIN_RD0_RD1_SET(reg,val) BSP_FLD32SET(reg, val,16, 23) 449 #define TMS570_LIN_RD0_RD2(val) BSP_FLD32(val,8, 15) 450 #define TMS570_LIN_RD0_RD2_GET(reg) BSP_FLD32GET(reg,8, 15) 451 #define TMS570_LIN_RD0_RD2_SET(reg,val) BSP_FLD32SET(reg, val,8, 15) 454 #define TMS570_LIN_RD0_RD3(val) BSP_FLD32(val,0, 7) 455 #define TMS570_LIN_RD0_RD3_GET(reg) BSP_FLD32GET(reg,0, 7) 456 #define TMS570_LIN_RD0_RD3_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 461 #define TMS570_LIN_RD1_RD4(val) BSP_FLD32(val,24, 31) 462 #define TMS570_LIN_RD1_RD4_GET(reg) BSP_FLD32GET(reg,24, 31) 463 #define TMS570_LIN_RD1_RD4_SET(reg,val) BSP_FLD32SET(reg, val,24, 31) 466 #define TMS570_LIN_RD1_RD5(val) BSP_FLD32(val,16, 23) 467 #define TMS570_LIN_RD1_RD5_GET(reg) BSP_FLD32GET(reg,16, 23) 468 #define TMS570_LIN_RD1_RD5_SET(reg,val) BSP_FLD32SET(reg, val,16, 23) 471 #define TMS570_LIN_RD1_RD6(val) BSP_FLD32(val,8, 15) 472 #define TMS570_LIN_RD1_RD6_GET(reg) BSP_FLD32GET(reg,8, 15) 473 #define TMS570_LIN_RD1_RD6_SET(reg,val) BSP_FLD32SET(reg, val,8, 15) 476 #define TMS570_LIN_RD1_RD7(val) BSP_FLD32(val,0, 7) 477 #define TMS570_LIN_RD1_RD7_GET(reg) BSP_FLD32GET(reg,0, 7) 478 #define TMS570_LIN_RD1_RD7_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 483 #define TMS570_LIN_MASK_RX_ID_MASK(val) BSP_FLD32(val,16, 23) 484 #define TMS570_LIN_MASK_RX_ID_MASK_GET(reg) BSP_FLD32GET(reg,16, 23) 485 #define TMS570_LIN_MASK_RX_ID_MASK_SET(reg,val) BSP_FLD32SET(reg, val,16, 23) 488 #define TMS570_LIN_MASK_TX_ID_MASK(val) BSP_FLD32(val,0, 7) 489 #define TMS570_LIN_MASK_TX_ID_MASK_GET(reg) BSP_FLD32GET(reg,0, 7) 490 #define TMS570_LIN_MASK_TX_ID_MASK_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 495 #define TMS570_LIN_ID_RECEIVED_ID(val) BSP_FLD32(val,16, 23) 496 #define TMS570_LIN_ID_RECEIVED_ID_GET(reg) BSP_FLD32GET(reg,16, 23) 497 #define TMS570_LIN_ID_RECEIVED_ID_SET(reg,val) BSP_FLD32SET(reg, val,16, 23) 500 #define TMS570_LIN_ID_ID_SLAVETASK_BYTE(val) BSP_FLD32(val,8, 15) 501 #define TMS570_LIN_ID_ID_SLAVETASK_BYTE_GET(reg) BSP_FLD32GET(reg,8, 15) 502 #define TMS570_LIN_ID_ID_SLAVETASK_BYTE_SET(reg,val) BSP_FLD32SET(reg, val,8, 15) 505 #define TMS570_LIN_ID_ID_BYTE(val) BSP_FLD32(val,0, 7) 506 #define TMS570_LIN_ID_ID_BYTE_GET(reg) BSP_FLD32GET(reg,0, 7) 507 #define TMS570_LIN_ID_ID_BYTE_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 512 #define TMS570_LIN_TD0_TD0(val) BSP_FLD32(val,24, 31) 513 #define TMS570_LIN_TD0_TD0_GET(reg) BSP_FLD32GET(reg,24, 31) 514 #define TMS570_LIN_TD0_TD0_SET(reg,val) BSP_FLD32SET(reg, val,24, 31) 517 #define TMS570_LIN_TD0_TD1(val) BSP_FLD32(val,16, 23) 518 #define TMS570_LIN_TD0_TD1_GET(reg) BSP_FLD32GET(reg,16, 23) 519 #define TMS570_LIN_TD0_TD1_SET(reg,val) BSP_FLD32SET(reg, val,16, 23) 522 #define TMS570_LIN_TD0_TD2(val) BSP_FLD32(val,8, 15) 523 #define TMS570_LIN_TD0_TD2_GET(reg) BSP_FLD32GET(reg,8, 15) 524 #define TMS570_LIN_TD0_TD2_SET(reg,val) BSP_FLD32SET(reg, val,8, 15) 527 #define TMS570_LIN_TD0_TD3(val) BSP_FLD32(val,0, 7) 528 #define TMS570_LIN_TD0_TD3_GET(reg) BSP_FLD32GET(reg,0, 7) 529 #define TMS570_LIN_TD0_TD3_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 534 #define TMS570_LIN_TD1_TD4(val) BSP_FLD32(val,24, 31) 535 #define TMS570_LIN_TD1_TD4_GET(reg) BSP_FLD32GET(reg,24, 31) 536 #define TMS570_LIN_TD1_TD4_SET(reg,val) BSP_FLD32SET(reg, val,24, 31) 539 #define TMS570_LIN_TD1_TD5(val) BSP_FLD32(val,16, 23) 540 #define TMS570_LIN_TD1_TD5_GET(reg) BSP_FLD32GET(reg,16, 23) 541 #define TMS570_LIN_TD1_TD5_SET(reg,val) BSP_FLD32SET(reg, val,16, 23) 544 #define TMS570_LIN_TD1_TD6(val) BSP_FLD32(val,8, 15) 545 #define TMS570_LIN_TD1_TD6_GET(reg) BSP_FLD32GET(reg,8, 15) 546 #define TMS570_LIN_TD1_TD6_SET(reg,val) BSP_FLD32SET(reg, val,8, 15) 549 #define TMS570_LIN_TD1_TD7(val) BSP_FLD32(val,0, 7) 550 #define TMS570_LIN_TD1_TD7_GET(reg) BSP_FLD32GET(reg,0, 7) 551 #define TMS570_LIN_TD1_TD7_SET(reg,val) BSP_FLD32SET(reg, val,0, 7) 556 #define TMS570_LIN_MBRSR_MBR(val) BSP_FLD32(val,0, 12) 557 #define TMS570_LIN_MBRSR_MBR_GET(reg) BSP_FLD32GET(reg,0, 12) 558 #define TMS570_LIN_MBRSR_MBR_SET(reg,val) BSP_FLD32SET(reg, val,0, 12) 563 #define TMS570_LIN_IODFTCTRL_FEN BSP_BIT32(26) 566 #define TMS570_LIN_IODFTCTRL_PEN BSP_BIT32(25) 569 #define TMS570_LIN_IODFTCTRL_BRKD_TENA BSP_BIT32(24) 572 #define TMS570_LIN_IODFTCTRL_PIN_SAMPLE_MASK(val) BSP_FLD32(val,19, 20) 573 #define TMS570_LIN_IODFTCTRL_PIN_SAMPLE_MASK_GET(reg) BSP_FLD32GET(reg,19, 20) 574 #define TMS570_LIN_IODFTCTRL_PIN_SAMPLE_MASK_SET(reg,val) BSP_FLD32SET(reg, val,19, 20) 577 #define TMS570_LIN_IODFTCTRL_TX_SHIFT(val) BSP_FLD32(val,16, 18) 578 #define TMS570_LIN_IODFTCTRL_TX_SHIFT_GET(reg) BSP_FLD32GET(reg,16, 18) 579 #define TMS570_LIN_IODFTCTRL_TX_SHIFT_SET(reg,val) BSP_FLD32SET(reg, val,16, 18) 582 #define TMS570_LIN_IODFTCTRL_IODFTENA(val) BSP_FLD32(val,8, 11) 583 #define TMS570_LIN_IODFTCTRL_IODFTENA_GET(reg) BSP_FLD32GET(reg,8, 11) 584 #define TMS570_LIN_IODFTCTRL_IODFTENA_SET(reg,val) BSP_FLD32SET(reg, val,8, 11) 587 #define TMS570_LIN_IODFTCTRL_LPBENA BSP_BIT32(1) 590 #define TMS570_LIN_IODFTCTRL_RXPENA BSP_BIT32(0)