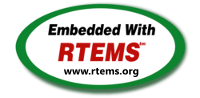 |
RTEMS
5.1
|
Go to the documentation of this file. 21 #ifndef LIBBSP_ARM_RASPBERRYPI_RASPBERRYPI_H 22 #define LIBBSP_ARM_RASPBERRYPI_RASPBERRYPI_H 44 #define BCM2835_REG(x) (*(volatile uint32_t *)(x)) 45 #define BCM2835_BIT(n) (1 << (n)) 55 #if (BSP_IS_RPI2 == 1) 56 #define RPI_PERIPHERAL_BASE 0x3F000000 57 #define BASE_OFFSET 0X3F000000 59 #define RPI_PERIPHERAL_BASE 0x20000000 60 #define BASE_OFFSET 0X5E000000 63 #define RPI_PERIPHERAL_SIZE 0x01000000 71 #define BUS_TO_PHY(x) ((x) - BASE_OFFSET) 81 #define BCM2835_CLOCK_FREQ 250000000 83 #define BCM2835_TIMER_BASE (RPI_PERIPHERAL_BASE + 0xB400) 85 #define BCM2835_TIMER_LOD (BCM2835_TIMER_BASE + 0x00) 86 #define BCM2835_TIMER_VAL (BCM2835_TIMER_BASE + 0x04) 87 #define BCM2835_TIMER_CTL (BCM2835_TIMER_BASE + 0x08) 88 #define BCM2835_TIMER_CLI (BCM2835_TIMER_BASE + 0x0C) 89 #define BCM2835_TIMER_RIS (BCM2835_TIMER_BASE + 0x10) 90 #define BCM2835_TIMER_MIS (BCM2835_TIMER_BASE + 0x14) 91 #define BCM2835_TIMER_RLD (BCM2835_TIMER_BASE + 0x18) 92 #define BCM2835_TIMER_DIV (BCM2835_TIMER_BASE + 0x1C) 93 #define BCM2835_TIMER_CNT (BCM2835_TIMER_BASE + 0x20) 95 #define BCM2835_TIMER_PRESCALE 0xF9 105 #define BCM2835_PM_PASSWD_MAGIC 0x5a000000 107 #define BCM2835_PM_BASE (RPI_PERIPHERAL_BASE + 0x100000) 109 #define BCM2835_PM_GNRIC (BCM2835_PM_BASE + 0x00) 110 #define BCM2835_PM_GNRIC_POWUP 0x00000001 111 #define BCM2835_PM_GNRIC_POWOK 0x00000002 112 #define BCM2835_PM_GNRIC_ISPOW 0x00000004 113 #define BCM2835_PM_GNRIC_MEMREP 0x00000008 114 #define BCM2835_PM_GNRIC_MRDONE 0x00000010 115 #define BCM2835_PM_GNRIC_ISFUNC 0x00000020 116 #define BCM2835_PM_GNRIC_RSTN 0x00000fc0 117 #define BCM2835_PM_GNRIC_ENAB 0x00001000 118 #define BCM2835_PM_GNRIC_CFG 0x007f0000 120 #define BCM2835_PM_AUDIO (BCM2835_PM_BASE + 0x04) 121 #define BCM2835_PM_AUDIO_APSM 0x000fffff 122 #define BCM2835_PM_AUDIO_CTRLEN 0x00100000 123 #define BCM2835_PM_AUDIO_RSTN 0x00200000 125 #define BCM2835_PM_STATUS (BCM2835_PM_BASE + 0x18) 127 #define BCM2835_PM_RSTC (BCM2835_PM_BASE + 0x1c) 128 #define BCM2835_PM_RSTC_DRCFG 0x00000003 129 #define BCM2835_PM_RSTC_WRCFG 0x00000030 130 #define BCM2835_PM_RSTC_WRCFG_FULL 0x00000020 131 #define BCM2835_PM_RSTC_SRCFG 0x00000300 132 #define BCM2835_PM_RSTC_QRCFG 0x00003000 133 #define BCM2835_PM_RSTC_FRCFG 0x00030000 134 #define BCM2835_PM_RSTC_HRCFG 0x00300000 136 #define BCM2835_PM_RSTS (BCM2835_PM_BASE + 0x20) 137 #define BCM2835_PM_RSTS_HADDRQ 0x00000001 138 #define BCM2835_PM_RSTS_HADDRF 0x00000002 139 #define BCM2835_PM_RSTS_HADDRH 0x00000004 140 #define BCM2835_PM_RSTS_HADWRQ 0x00000010 141 #define BCM2835_PM_RSTS_HADWRF 0x00000020 142 #define BCM2835_PM_RSTS_HADWRH 0x00000040 143 #define BCM2835_PM_RSTS_HADSRQ 0x00000100 144 #define BCM2835_PM_RSTS_HADSRF 0x00000200 145 #define BCM2835_PM_RSTS_HADSRH 0x00000400 146 #define BCM2835_PM_RSTS_HADPOR 0x00001000 148 #define BCM2835_PM_WDOG (BCM2835_PM_BASE + 0x24) 158 #define BCM2835_GPIO_REGS_BASE (RPI_PERIPHERAL_BASE + 0x200000) 160 #define BCM2835_GPIO_GPFSEL1 (BCM2835_GPIO_REGS_BASE + 0x04) 161 #define BCM2835_GPIO_GPSET0 (BCM2835_GPIO_REGS_BASE + 0x1C) 162 #define BCM2835_GPIO_GPCLR0 (BCM2835_GPIO_REGS_BASE + 0x28) 163 #define BCM2835_GPIO_GPLEV0 (BCM2835_GPIO_REGS_BASE + 0x34) 164 #define BCM2835_GPIO_GPEDS0 (BCM2835_GPIO_REGS_BASE + 0x40) 165 #define BCM2835_GPIO_GPREN0 (BCM2835_GPIO_REGS_BASE + 0x4C) 166 #define BCM2835_GPIO_GPFEN0 (BCM2835_GPIO_REGS_BASE + 0x58) 167 #define BCM2835_GPIO_GPHEN0 (BCM2835_GPIO_REGS_BASE + 0x64) 168 #define BCM2835_GPIO_GPLEN0 (BCM2835_GPIO_REGS_BASE + 0x70) 169 #define BCM2835_GPIO_GPAREN0 (BCM2835_GPIO_REGS_BASE + 0x7C) 170 #define BCM2835_GPIO_GPAFEN0 (BCM2835_GPIO_REGS_BASE + 0x88) 171 #define BCM2835_GPIO_GPPUD (BCM2835_GPIO_REGS_BASE + 0x94) 172 #define BCM2835_GPIO_GPPUDCLK0 (BCM2835_GPIO_REGS_BASE + 0x98) 182 #define BCM2835_AUX_BASE (RPI_PERIPHERAL_BASE + 0x215000) 184 #define AUX_ENABLES (BCM2835_AUX_BASE + 0x04) 185 #define AUX_MU_IO_REG (BCM2835_AUX_BASE + 0x40) 186 #define AUX_MU_IER_REG (BCM2835_AUX_BASE + 0x44) 187 #define AUX_MU_IIR_REG (BCM2835_AUX_BASE + 0x48) 188 #define AUX_MU_LCR_REG (BCM2835_AUX_BASE + 0x4C) 189 #define AUX_MU_MCR_REG (BCM2835_AUX_BASE + 0x50) 190 #define AUX_MU_LSR_REG (BCM2835_AUX_BASE + 0x54) 191 #define AUX_MU_MSR_REG (BCM2835_AUX_BASE + 0x58) 192 #define AUX_MU_SCRATCH (BCM2835_AUX_BASE + 0x5C) 193 #define AUX_MU_CNTL_REG (BCM2835_AUX_BASE + 0x60) 194 #define AUX_MU_STAT_REG (BCM2835_AUX_BASE + 0x64) 195 #define AUX_MU_BAUD_REG (BCM2835_AUX_BASE + 0x68) 207 #define BCM2835_I2C_BASE (RPI_PERIPHERAL_BASE + 0x804000) 209 #define BCM2835_I2C_C (BCM2835_I2C_BASE + 0x00) 210 #define BCM2835_I2C_S (BCM2835_I2C_BASE + 0x04) 211 #define BCM2835_I2C_DLEN (BCM2835_I2C_BASE + 0x08) 212 #define BCM2835_I2C_A (BCM2835_I2C_BASE + 0x0C) 213 #define BCM2835_I2C_FIFO (BCM2835_I2C_BASE + 0x10) 214 #define BCM2835_I2C_DIV (BCM2835_I2C_BASE + 0x14) 215 #define BCM2835_I2C_DEL (BCM2835_I2C_BASE + 0x18) 216 #define BCM2835_I2C_CLKT (BCM2835_I2C_BASE + 0x1C) 226 #define BCM2835_SPI_BASE (RPI_PERIPHERAL_BASE + 0x204000) 228 #define BCM2835_SPI_CS (BCM2835_SPI_BASE + 0x00) 229 #define BCM2835_SPI_FIFO (BCM2835_SPI_BASE + 0x04) 230 #define BCM2835_SPI_CLK (BCM2835_SPI_BASE + 0x08) 231 #define BCM2835_SPI_DLEN (BCM2835_SPI_BASE + 0x0C) 232 #define BCM2835_SPI_LTOH (BCM2835_SPI_BASE + 0x10) 233 #define BCM2835_SPI_DC (BCM2835_SPI_BASE + 0x14) 243 #define BCM2835_I2C_SPI_BASE (RPI_PERIPHERAL_BASE + 0x214000) 245 #define BCM2835_I2C_SPI_DR (BCM2835_I2C_SPI_BASE + 0x00) 246 #define BCM2835_I2C_SPI_RSR (BCM2835_I2C_SPI_BASE + 0x04) 247 #define BCM2835_I2C_SPI_SLV (BCM2835_I2C_SPI_BASE + 0x08) 248 #define BCM2835_I2C_SPI_CR (BCM2835_I2C_SPI_BASE + 0x0C) 249 #define BCM2835_I2C_SPI_FR (BCM2835_I2C_SPI_BASE + 0x10) 250 #define BCM2835_I2C_SPI_IFLS (BCM2835_I2C_SPI_BASE + 0x14) 251 #define BCM2835_I2C_SPI_IMSC (BCM2835_I2C_SPI_BASE + 0x18) 252 #define BCM2835_I2C_SPI_RIS (BCM2835_I2C_SPI_BASE + 0x1C) 253 #define BCM2835_I2C_SPI_MIS (BCM2835_I2C_SPI_BASE + 0x20) 254 #define BCM2835_I2C_SPI_ICR (BCM2835_I2C_SPI_BASE + 0x24) 255 #define BCM2835_I2C_SPI_DMACR (BCM2835_I2C_SPI_BASE + 0x28) 256 #define BCM2835_I2C_SPI_TDR (BCM2835_I2C_SPI_BASE + 0x2C) 257 #define BCM2835_I2C_SPI_GPUSTAT (BCM2835_I2C_SPI_BASE + 0x30) 258 #define BCM2835_I2C_SPI_HCTRL (BCM2835_I2C_SPI_BASE + 0x34) 268 #define BCM2835_BASE_INTC (RPI_PERIPHERAL_BASE + 0xB200) 270 #define BCM2835_IRQ_BASIC (BCM2835_BASE_INTC + 0x00) 271 #define BCM2835_IRQ_PENDING1 (BCM2835_BASE_INTC + 0x04) 272 #define BCM2835_IRQ_PENDING2 (BCM2835_BASE_INTC + 0x08) 273 #define BCM2835_IRQ_FIQ_CTRL (BCM2835_BASE_INTC + 0x0C) 274 #define BCM2835_IRQ_ENABLE1 (BCM2835_BASE_INTC + 0x10) 275 #define BCM2835_IRQ_ENABLE2 (BCM2835_BASE_INTC + 0x14) 276 #define BCM2835_IRQ_ENABLE_BASIC (BCM2835_BASE_INTC + 0x18) 277 #define BCM2835_IRQ_DISABLE1 (BCM2835_BASE_INTC + 0x1C) 278 #define BCM2835_IRQ_DISABLE2 (BCM2835_BASE_INTC + 0x20) 279 #define BCM2835_IRQ_DISABLE_BASIC (BCM2835_BASE_INTC + 0x24) 294 #define BCM2835_GPU_TIMER_BASE (RPI_PERIPHERAL_BASE + 0x3000) 296 #define BCM2835_GPU_TIMER_CS (BCM2835_GPU_TIMER_BASE + 0x00) 297 #define BCM2835_GPU_TIMER_CS_M0 0x00000001 298 #define BCM2835_GPU_TIMER_CS_M1 0x00000002 299 #define BCM2835_GPU_TIMER_CS_M2 0x00000004 300 #define BCM2835_GPU_TIMER_CS_M3 0x00000008 301 #define BCM2835_GPU_TIMER_CLO (BCM2835_GPU_TIMER_BASE + 0x04) 302 #define BCM2835_GPU_TIMER_CHI (BCM2835_GPU_TIMER_BASE + 0x08) 303 #define BCM2835_GPU_TIMER_C0 (BCM2835_GPU_TIMER_BASE + 0x0C) 304 #define BCM2835_GPU_TIMER_C1 (BCM2835_GPU_TIMER_BASE + 0x10) 305 #define BCM2835_GPU_TIMER_C2 (BCM2835_GPU_TIMER_BASE + 0x14) 306 #define BCM2835_GPU_TIMER_C3 (BCM2835_GPU_TIMER_BASE + 0x18) 321 #define BCM2835_EMMC_BASE (RPI_PERIPHERAL_BASE + 0x300000) 331 #define BCM2835_MBOX_BASE (RPI_PERIPHERAL_BASE+0xB880) 333 #define BCM2835_MBOX_PEEK (BCM2835_MBOX_BASE+0x10) 334 #define BCM2835_MBOX_READ (BCM2835_MBOX_BASE+0x00) 335 #define BCM2835_MBOX_WRITE (BCM2835_MBOX_BASE+0x20) 336 #define BCM2835_MBOX_STATUS (BCM2835_MBOX_BASE+0x18) 337 #define BCM2835_MBOX_SENDER (BCM2835_MBOX_BASE+0x14) 338 #define BCM2835_MBOX_CONFIG (BCM2835_MBOX_BASE+0x1C) 340 #define BCM2835_MBOX_FULL 0x80000000 341 #define BCM2835_MBOX_EMPTY 0x40000000 352 #define BCM2835_MBOX_CHANNEL_PM 0 354 #define BCM2835_MBOX_CHANNEL_FB 1 356 #define BCM2835_MBOX_CHANNEL_VUART 2 358 #define BCM2835_MBOX_CHANNEL_VCHIQ 3 360 #define BCM2835_MBOX_CHANNEL_LED 4 362 #define BCM2835_MBOX_CHANNEL_BUTTON 5 364 #define BCM2835_MBOX_CHANNEL_TOUCHS 6 366 #define BCM2835_MBOX_CHANNEL_PROP_AVC 8 368 #define BCM2835_MBOX_CHANNEL_PROP_VCA 9 378 #define BCM2835_USB_BASE (RPI_PERIPHERAL_BASE + 0x980000) 388 #define BCM2836_CORE_LOCAL_PERIPH_BASE 0x40000000 389 #define BCM2836_CORE_LOCAL_PERIPH_SIZE 0x00040000 399 #define BCM2836_MAILBOX_0_WRITE_SET_BASE 0x40000080 400 #define BCM2836_MAILBOX_1_WRITE_SET_BASE 0x40000084 401 #define BCM2836_MAILBOX_2_WRITE_SET_BASE 0x40000088 402 #define BCM2836_MAILBOX_3_WRITE_SET_BASE 0x4000008C 403 #define BCM2836_MAILBOX_0_READ_CLEAR_BASE 0x400000C0 404 #define BCM2836_MAILBOX_1_READ_CLEAR_BASE 0x400000C4 405 #define BCM2836_MAILBOX_2_READ_CLEAR_BASE 0x400000C8 406 #define BCM2836_MAILBOX_3_READ_CLEAR_BASE 0x400000CC 416 #define BCM2836_CORE_TIMER_CTRL 0x40000000 418 #define BCM2836_CORE_TIMER_CTRL_APB_CLK 0x00000100 419 #define BCM2836_CORE_TIMER_CTRL_INC_2 0x00000200 421 #define BCM2836_CORE_TIMER_PRESCALER 0x40000008 423 #define BCM2836_CORE_TIMER_LS32 0x4000001C 424 #define BCM2836_CORE_TIMER_MS32 0x40000020 434 #define BCM2836_LOCAL_TIMER_CTRL 0x40000034 436 #define BCM2836_LOCAL_TIMER_CTRL_IRQ_FLAG 0x80000000 437 #define BCM2836_LOCAL_TIMER_CTRL_IRQ_EN 0x20000000 438 #define BCM2836_LOCAL_TIMER_CTRL_TIMER_EN 0x10000000 439 #define BCM2836_LOCAL_TIMER_RELOAD 0x0FFFFFFF 441 #define BCM2836_LOCAL_TIMER_IRQ_RELOAD 0x40000038 443 #define BCM2836_LOCAL_TIMER_IRQ_CLEAR 0x80000000 444 #define BCM2836_LOCAL_TIMER_RELOAD_NOW 0x40000000 446 #define BCM2836_LOCAL_TIMER_IRQ_ROUTING 0x40000024 447 #define BCM2836_LOCAL_TIMER_ROU_CORE0_IRQ 0x00 448 #define BCM2836_LOCAL_TIMER_ROU_CORE1_IRQ 0x01 449 #define BCM2836_LOCAL_TIMER_ROU_CORE2_IRQ 0x02 450 #define BCM2836_LOCAL_TIMER_ROU_CORE3_IRQ 0x03 451 #define BCM2836_LOCAL_TIMER_ROU_CORE0_FIQ 0x04 452 #define BCM2836_LOCAL_TIMER_ROU_CORE1_FIQ 0x05 453 #define BCM2836_LOCAL_TIMER_ROU_CORE2_FIQ 0x06 454 #define BCM2836_LOCAL_TIMER_ROU_CORE3_FIQ 0x07 464 #define BCM2836_GPU_IRQ_ROUTING 0x4000000C 466 #define BCM2836_GPU_IRQ_ROU_GPU_IRQ_CORE0 0x00000000 467 #define BCM2836_GPU_IRQ_ROU_GPU_IRQ_CORE1 0x00000001 468 #define BCM2836_GPU_IRQ_ROU_GPU_IRQ_CORE2 0x00000002 469 #define BCM2836_GPU_IRQ_ROU_GPU_IRQ_CORE4 0x00000003 471 #define BCM2836_GPU_IRQ_ROU_GPU_FIQ_CORE0 0x00000000 472 #define BCM2836_GPU_IRQ_ROU_GPU_FIQ_CORE1 0x00000004 473 #define BCM2836_GPU_IRQ_ROU_GPU_FIQ_CORE2 0x00000008 474 #define BCM2836_GPU_IRQ_ROU_GPU_FIQ_CORE4 0x0000000C 476 #define BCM2836_GPU_IRQ_ROU_GPU_FIQ_CORE4 0x0000000C 488 #define BCM2836_CORE0_TIMER_IRQ_CTRL_BASE 0x40000040 489 #define BCM2836_CORE1_TIMER_IRQ_CTRL_BASE 0x40000044 490 #define BCM2836_CORE2_TIMER_IRQ_CTRL_BASE 0x40000048 491 #define BCM2836_CORE3_TIMER_IRQ_CTRL_BASE 0x4000004C 493 #define BCM2836_CORE_TIMER_IRQ_CTRL(cpuidx) \ 494 (BCM2836_CORE0_TIMER_IRQ_CTRL_BASE + 0x4 * (cpuidx)) 500 #define BCM2836_CORE_TIMER_IRQ_CTRL_TIMER0_IRQ 0x01 501 #define BCM2836_CORE_TIMER_IRQ_CTRL_TIMER1_IRQ 0x02 502 #define BCM2836_CORE_TIMER_IRQ_CTRL_TIMER2_IRQ 0x04 503 #define BCM2836_CORE_TIMER_IRQ_CTRL_TIMER3_IRQ 0x08 504 #define BCM2836_CORE_TIMER_IRQ_CTRL_TIMER0_FIQ 0x10 505 #define BCM2836_CORE_TIMER_IRQ_CTRL_TIMER1_FIQ 0x20 506 #define BCM2836_CORE_TIMER_IRQ_CTRL_TIMER2_FIQ 0x40 507 #define BCM2836_CORE_TIMER_IRQ_CTRL_TIMER3_FIQ 0x80 510 #define BCM2836_MAILBOX_IRQ_CTRL_BASE 0x40000050 511 #define BCM2836_MAILBOX_IRQ_CTRL(cpuidx) \ 512 (BCM2836_MAILBOX_IRQ_CTRL_BASE + 0x4 * (cpuidx)) 517 #define BCM2836_MAILBOX_IRQ_CTRL_MBOX0_IRQ 0x01 518 #define BCM2836_MAILBOX_IRQ_CTRL_MBOX1_IRQ 0x02 519 #define BCM2836_MAILBOX_IRQ_CTRL_MBOX2_IRQ 0x04 520 #define BCM2836_MAILBOX_IRQ_CTRL_MBOX3_IRQ 0x08 521 #define BCM2836_MAILBOX_IRQ_CTRL_MBOX0_FIQ 0x10 522 #define BCM2836_MAILBOX_IRQ_CTRL_MBOX1_FIQ 0x20 523 #define BCM2836_MAILBOX_IRQ_CTRL_MBOX2_FIQ 0x40 524 #define BCM2836_MAILBOX_IRQ_CTRL_MBOX3_FIQ 0x80 526 #define BCM2836_IRQ_SOURCE_REG_BASE 0x40000060 527 #define BCM2836_IRQ_SOURCE_REG(cpuidx) \ 528 (BCM2836_IRQ_SOURCE_REG_BASE + 0x4 * (cpuidx)) 530 #define BCM2836_FIQ_SOURCE_REG_BASE 0x40000070 531 #define BCM2836_FIQ_SOURCE_REG(cpuidx) \ 532 (BCM2836_FIQ_SOURCE_REG_BASE + 0x4 * (cpuidx)) 534 #define BCM2836_IRQ_SOURCE_TIMER0 0x00000001 535 #define BCM2836_IRQ_SOURCE_TIMER1 0x00000002 536 #define BCM2836_IRQ_SOURCE_TIMER2 0x00000004 537 #define BCM2836_IRQ_SOURCE_TIMER3 0x00000008 538 #define BCM2836_IRQ_SOURCE_MBOX0 0x00000010 539 #define BCM2836_IRQ_SOURCE_MBOX1 0x00000020 540 #define BCM2836_IRQ_SOURCE_MBOX2 0x00000040 541 #define BCM2836_IRQ_SOURCE_MBOX3 0x00000080 542 #define BCM2836_IRQ_SOURCE_GPU 0x00000100 543 #define BCM2836_IRQ_SOURCE_PMU 0x00000200 544 #define BCM2836_IRQ_SOURCE_LOCAL_TIMER 0x00000800