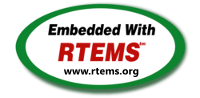 |
RTEMS
5.1
|
12 typedef unsigned int word_t;
16 #define PRIMARY_IRQS 32 17 #define GPIO_IRQS (85 - 2) 21 #define IRQS (PRIMARY_IRQS + GPIO_IRQS) 24 #define INTERRUPT_OFFSET 0xd00000 25 #define XSCALE_IRQ_OS_TIMER 26 26 #define XSCALE_IRQ_PMU 12 27 #define XSCALE_IRQ_STUART 20 28 #define XSCALE_IRQ_NETWORK 16 31 #define CCNT_IRQ_ENABLE 1UL << 6 32 #define PMN1_IRQ_ENABLE 1UL << 5 33 #define PMN0_IRQ_ENABLE 1UL << 4 35 #define IODEVICE_VADDR 0x40000000 36 #define XSCALE_INT (IODEVICE_VADDR + INTERRUPT_OFFSET) 38 #define XSCALE_INT_ICMR (*(volatile word_t *)(XSCALE_INT + 0x04)) 39 #define XSCALE_INT_ICLR (*(volatile word_t *)(XSCALE_INT + 0x08)) 40 #define XSCALE_INT_ICCR (*(volatile word_t *)(XSCALE_INT + 0x14)) 41 #define XSCALE_INT_ICIP (*(volatile word_t *)(XSCALE_INT + 0x00)) 42 #define XSCALE_INT_ICFP (*(volatile word_t *)(XSCALE_INT + 0x0c)) 43 #define XSCALE_INT_ICPR (*(volatile word_t *)(XSCALE_INT + 0x10)) 46 #define GPIO_OFFSET 0xe00000 47 #define PXA_GPIO (IODEVICE_VADDR + GPIO_OFFSET) 49 #define PXA_GEDR0 (*(volatile word_t *)(PXA_GPIO + 0x48)) 50 #define PXA_GEDR1 (*(volatile word_t *)(PXA_GPIO + 0x4C)) 51 #define PXA_GEDR2 (*(volatile word_t *)(PXA_GPIO + 0x50)) 56 #define TIMER_OFFSET 0x0a00000 57 #define CLOCKS_OFFSET 0x1300000 60 #define TIMER_RATE 36864 62 #define XSCALE_TIMERS (IODEVICE_VADDR + TIMER_OFFSET) 65 #define XSCALE_OS_TIMER_MR0 (*(volatile word_t *)(XSCALE_TIMERS + 0x00)) 66 #define XSCALE_OS_TIMER_MR1 (*(volatile word_t *)(XSCALE_TIMERS + 0x04)) 67 #define XSCALE_OS_TIMER_MR2 (*(volatile word_t *)(XSCALE_TIMERS + 0x08)) 68 #define XSCALE_OS_TIMER_MR3 (*(volatile word_t *)(XSCALE_TIMERS + 0x0c)) 71 #define XSCALE_OS_TIMER_IER (*(volatile word_t *)(XSCALE_TIMERS + 0x1c)) 73 #define XSCALE_OS_TIMER_WMER (*(volatile word_t *)(XSCALE_TIMERS + 0x18)) 75 #define XSCALE_OS_TIMER_TCR (*(volatile word_t *)(XSCALE_TIMERS + 0x10)) 77 #define XSCALE_OS_TIMER_TSR (*(volatile word_t *)(XSCALE_TIMERS + 0x14)) 79 #define XSCALE_CLOCKS (IODEVICE_VADDR + CLOCKS_VOFFSET) 81 #define XSCALE_CLOCKS_CCCR (*(volatile word_t *)(XSCALE_CLOCKS + 0x00)) 84 #define FFUART_BASE 0x40100000 88 #define SKYEYE_MAGIC_ADDRESS (*(volatile word_t *)(0xb0000000)) 89 #define SKYEYE_MAGIC_NUMBER (0xf0f0f0f0) 102 #define PMC_PMNC_E (0x01) 103 #define PMC_PMNC_PCR (0x01 << 1) 104 #define PMC_PMNC_CCR (0x01 << 2) 105 #define PMC_PMNC_CCD (0x01 << 3) 106 #define PMC_PMNC_PCD (0x01 << 4) 109 #define LCCR0 (*(volatile word_t *)(0x44000000)) 110 #define LCCR1 (*(volatile word_t *)(0x44000004)) 111 #define LCCR2 (*(volatile word_t *)(0x44000008)) 112 #define LCCR3 (*(volatile word_t *)(0x4400000C)) 114 #define FDADR0 (*(volatile word_t *)(0x44000200)) 115 #define FSADR0 (*(volatile word_t *)(0x44000204)) 116 #define FIDR0 (*(volatile word_t *)(0x44000208)) 117 #define LDCMD0 (*(volatile word_t *)(0x4400020C)) 119 #define FDADR1 (*(volatile word_t *)(0x44000210)) 120 #define FSADR1 (*(volatile word_t *)(0x44000214)) 121 #define FIDR1 (*(volatile word_t *)(0x44000218)) 122 #define LDCMD1 (*(volatile word_t *)(0x4400021C)) 124 #define LCCR0_ENB 0x00000001 125 #define LCCR1_PPL 0x000003FF 126 #define LCCR2_LPP 0x000003FF 127 #define LCCR3_BPP 0x07000000