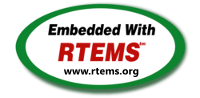 |
RTEMS
5.1
|
Go to the documentation of this file. 65 #define ALT_E_SUCCESS 0 68 #define ALT_E_ERROR (-1) 70 #define ALT_E_FPGA_CFG (-2) 72 #define ALT_E_FPGA_CRC (-3) 74 #define ALT_E_FPGA_CFG_STM (-4) 76 #define ALT_E_FPGA_PWR_OFF (-5) 78 #define ALT_E_FPGA_NO_SOC_CTRL (-6) 80 #define ALT_E_FPGA_NOT_USER_MODE (-7) 82 #define ALT_E_ARG_RANGE (-8) 84 #define ALT_E_BAD_ARG (-9) 86 #define ALT_E_BAD_OPERATION (-10) 88 #define ALT_E_INV_OPTION (-11) 90 #define ALT_E_TMO (-12) 92 #define ALT_E_RESERVED (-13) 94 #define ALT_E_BAD_CLK (-14) 96 #define ALT_E_BAD_VERSION (-15) 98 #define ALT_E_BUF_OVF (-20) 103 #define ALT_E_FALSE (0) 107 #define ALT_E_TRUE (1) 132 #define ALT_HWLIBS_VERSION(a,b,c) (((a)*10000)+((b)*100)+(c)) 134 #define ALTERA_HWLIBS_VERSION_CODE ALT_HWLIBS_VERSION(ALTERA_ACDS_MAJOR_REV, \ 135 ALTERA_ACDS_MINOR_REV, ALTERA_HWLIBS_REV) 140 #define ALTERA_INTERNAL_ONLY_DOCS 1 148 #define ALT_HPS_ADDR 0 154 #define ALT_MILLISECS_IN_A_SEC 1000 155 #define ALT_MICROSECS_IN_A_SEC 1000000 156 #define ALT_NANOSECS_IN_A_SEC 1000000000 158 #define ALT_TWO_TO_POW0 (1) 159 #define ALT_TWO_TO_POW1 (1<<1) 160 #define ALT_TWO_TO_POW2 (1<<2) 161 #define ALT_TWO_TO_POW3 (1<<3) 162 #define ALT_TWO_TO_POW4 (1<<4) 163 #define ALT_TWO_TO_POW5 (1<<5) 164 #define ALT_TWO_TO_POW6 (1<<6) 165 #define ALT_TWO_TO_POW7 (1<<7) 166 #define ALT_TWO_TO_POW8 (1<<8) 167 #define ALT_TWO_TO_POW9 (1<<9) 168 #define ALT_TWO_TO_POW10 (1<<10) 169 #define ALT_TWO_TO_POW11 (1<<11) 170 #define ALT_TWO_TO_POW12 (1<<12) 171 #define ALT_TWO_TO_POW13 (1<<13) 172 #define ALT_TWO_TO_POW14 (1<<14) 173 #define ALT_TWO_TO_POW15 (1<<15) 174 #define ALT_TWO_TO_POW16 (1<<16) 175 #define ALT_TWO_TO_POW17 (1<<17) 176 #define ALT_TWO_TO_POW18 (1<<18) 177 #define ALT_TWO_TO_POW19 (1<<19) 178 #define ALT_TWO_TO_POW20 (1<<20) 179 #define ALT_TWO_TO_POW21 (1<<21) 180 #define ALT_TWO_TO_POW22 (1<<22) 181 #define ALT_TWO_TO_POW23 (1<<23) 182 #define ALT_TWO_TO_POW24 (1<<24) 183 #define ALT_TWO_TO_POW25 (1<<25) 184 #define ALT_TWO_TO_POW26 (1<<26) 185 #define ALT_TWO_TO_POW27 (1<<27) 186 #define ALT_TWO_TO_POW28 (1<<28) 187 #define ALT_TWO_TO_POW29 (1<<29) 188 #define ALT_TWO_TO_POW30 (1<<30) 189 #define ALT_TWO_TO_POW31 (1<<31)
int32_t ALT_STATUS_CODE
Definition: hwlib.h:60