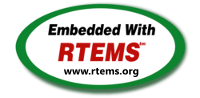 |
RTEMS
5.1
|
Go to the documentation of this file. 56 int (*func)(int,
char **);
69 #define CMDFLAG_NOMONRC 1 74 #define CMDLINESIZE 128 114 #define CMD_SUCCESS 0 115 #define CMD_FAILURE -1 116 #define CMD_PARAM_ERROR -2 117 #define CMD_LINE_ERROR -3 118 #define CMD_ULVL_DENIED -4 119 #define CMD_NOT_FOUND -5 120 #define CMD_MONRC_DENIED -6