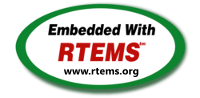 |
RTEMS
5.1
|
15 #ifndef BESTCOMM_OPS_H 16 #define BESTCOMM_OPS_H 36 #define INC(i) (24 + (i)) 38 #define IDX(i) (48 + (i)) 48 #define COND_FOREVER 7 50 #define INC_INIT(cond, val) \ 51 (BSP_FLD32(cond, 29, 31) \ 52 | BSP_FLD32((int16_t) (val), 0, 15)) 61 #define LCD_TERM(val) BSP_FLD32(val, 13, 14) 63 #define LCD(deref0, iniidx0, deref1, iniidx1, term, termop, inc0, inc1) \ 65 | BSP_FLD32(deref0, 29, 29) \ 66 | BSP_FLD32(iniidx0, 23, 28) \ 67 | BSP_FLD32(deref1, 21, 21) \ 68 | BSP_FLD32(iniidx1, 15, 20) \ 70 | BSP_FLD32(termop, 6, 11) \ 71 | BSP_FLD32(inc0, 3, 5) \ 72 | BSP_FLD32(inc1, 0, 2)) 74 #define LCDEXT(deref0, iniidx0, deref1, iniidx1, term, termop, inc0, inc1) \ 76 | LCD(deref0, iniidx0, deref1, iniidx1, term, termop, inc0, inc1)) 78 #define LCDPLUS(deref0, iniidx0, deref1, iniidx1, term, termop, inc0, inc1) \ 80 | LCD(deref0, iniidx0, deref1, iniidx1, term, termop, inc0, inc1)) 82 #define LCDINIT(val) \ 84 | BSP_FLD32((val) >> 13, 15, 29) \ 85 | LCD_TERM(TERM_INIT) \ 86 | BSP_FLD32(val, 0, 12)) 94 #define DRD_FLAGS(val) BSP_FLD32(val, 26, 28) 97 #define INIT_SCTMR_0 1 98 #define INIT_SCTMR_1 2 100 #define INIT_FEC_TX 4 101 #define INIT_ATA_RX 5 102 #define INIT_ATA_TX 6 103 #define INIT_SCPCI_RX 7 104 #define INIT_SCPCI_TX 8 105 #define INIT_PSC3_RX 9 106 #define INIT_PSC3_TX 10 107 #define INIT_PSC2_RX 11 108 #define INIT_PSC2_TX 12 109 #define INIT_PSC1_RX 13 110 #define INIT_PSC1_TX 14 111 #define INIT_SCTMR_2 15 112 #define INIT_SCLPC 16 113 #define INIT_PSC5_RX 17 114 #define INIT_PSC5_TX 18 115 #define INIT_PSC4_RX 19 116 #define INIT_PSC4_TX 20 117 #define INIT_I2C2_RX 21 118 #define INIT_I2C2_TX 22 119 #define INIT_I2C1_RX 23 120 #define INIT_I2C1_TX 24 121 #define INIT_PSC6_RX 25 122 #define INIT_PSC6_TX 26 123 #define INIT_IRDA_RX 25 124 #define INIT_IRDA_TX 26 125 #define INIT_SCTMR_3 27 126 #define INIT_SCTMR_4 28 127 #define INIT_SCTMR_5 29 128 #define INIT_SCTMR_6 30 129 #define INIT_SCTMR_7 31 131 #define DRD_INIT(val) BSP_FLD32(val, 21, 25) 138 #define DRD_RS(val) BSP_FLD32(val, 19, 20) 140 #define DRD_WS(val) BSP_FLD32(val, 17, 18) 142 #define DEST_VAR(val) (val) 143 #define DEST_IDX(val) (BSP_BIT32(5) | (val)) 144 #define DEST_DEREF_IDX(val) (BSP_BIT32(5) | BSP_BIT32(4) | (val)) 146 #define SRC_VAR(val) (val) 147 #define SRC_INC(val) (BSP_BIT32(5) | (val)) 148 #define SRC_EU_RESULT (BSP_BIT32(5) | BSP_BIT32(4) | BSP_BIT32(1) | BSP_BIT32(0)) 149 #define SRC_DEREF_EU_RESULT (BSP_BIT32(6) | BSP_BIT32(4) | BSP_BIT32(1) | BSP_BIT32(0)) 150 #define SRC_IDX(val) (BSP_BIT32(6) | BSP_BIT32(5) | (val)) 151 #define SRC_DEREF_IDX(val) (BSP_BIT32(6) | BSP_BIT32(5) | BSP_BIT32(4) | (val)) 152 #define SRC_NONE (BSP_BIT32(5) | BSP_BIT32(4) | BSP_BIT32(3) | BSP_BIT32(2) | BSP_BIT32(1) | BSP_BIT32(0)) 154 #define DRD1A(flags, init, dest, ws, src, rs) \ 159 | BSP_FLD32(dest, 10, 15) \ 160 | BSP_FLD32(src, 3, 9)) 162 #define DRD1AEURESULT(flags, init, dest, ws, rs) \ 163 (DRD1A(flags, init, rs, ws, dest, SRC_EU_RESULT) \ 164 | BSP_FLD32(1, 0, 3)) 166 #define FUNC_LOAD_ACC 0 167 #define FUNC_UNLOAD_ACC 1 178 #define FUNC_CRC16 12 179 #define FUNC_CRC32 13 180 #define FUNC_ENDIAN32 14 181 #define FUNC_ENDIAN16 15 183 #define DRD2A(flags, func) \ 184 (BSP_BIT32(30) | BSP_BIT32(29) \ 186 | BSP_FLD32(func, 0, 3)) 188 #define DRD2A5(flags, init, func, ws, rs) \ 189 (DRD2A(flags, func) \ 194 #define OP_VAR(val) (val) 195 #define OP_EU_RESULT (BSP_BIT32(4) | BSP_BIT32(3) | BSP_BIT32(1) | BSP_BIT32(0)) 196 #define OP_NONE (BSP_BIT32(4) | BSP_BIT32(3) | BSP_BIT32(2) | BSP_BIT32(1) | BSP_BIT32(0)) 197 #define OP_IDX(val) (BSP_BIT32(5) | (val)) 198 #define OP_DEREF_IDX(val) (BSP_BIT32(5) | BSP_BIT32(4) | (val)) 200 #define DRD2B1(dest, op0, op1) \ 201 (BSP_FLD32(dest, 22, 27) \ 202 | BSP_FLD32(SRC_EU_RESULT, 14, 20) \ 203 | BSP_FLD32(3, 12, 13) \ 204 | BSP_FLD32(op0, 6, 11) \ 205 | BSP_FLD32(op1, 0, 5)) 207 #define DRD2B2(op0, op1) \ 209 | BSP_FLD32(3, 26, 27) \ 210 | BSP_FLD32(op0, 20, 25) \ 211 | BSP_FLD32(op1, 14, 19) \ 212 | BSP_FLD32(0, 12, 13) \ 213 | BSP_FLD32(OP_NONE, 6, 11) \ 214 | BSP_FLD32(OP_NONE, 0, 5))