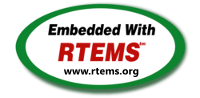 |
RTEMS
5.1
|
Go to the documentation of this file. 75 #define F_RX_FIRST 0x1 77 #define F_ACCESS_32_BITS 0x100 79 #define TX_INIT_RATE 16 80 #define TX_INIT_MAX_RATE 64 81 #define RX_INIT_LATENCY 64 82 #define RX_INIT_EARLY_THRESH 208 83 #define RX_NEXT_EARLY_THRESH 500 85 #define EEPROMSIZE 0x40 86 #define MAX_EEPROMBUSY 1000 87 #define EP_LAST_TAG 0xd7 88 #define EP_MAX_BOARDS 16 103 #define EP_ID_PORT 0x110 109 #define IS_BASE (is->id_iobase) 110 #define BASE (sc->ep_io_addr) 116 #define EEPROM_CMD_RD 0x0080 117 #define EEPROM_CMD_WR 0x0040 118 #define EEPROM_CMD_ERASE 0x00c0 119 #define EEPROM_CMD_EWEN 0x0030 121 #define EEPROM_BUSY (1<<15) 122 #define EEPROM_TST_MODE (1<<14) 127 #define is_eeprom_busy(b) (inw((b)+EP_W0_EEPROM_COMMAND)&EEPROM_BUSY) 128 #define GO_WINDOW(x) outw(BASE+EP_COMMAND, WINDOW_SELECT|(x)) 140 #define EEPROM_NODE_ADDR_0 0x0 141 #define EEPROM_NODE_ADDR_1 0x1 142 #define EEPROM_NODE_ADDR_2 0x2 143 #define EEPROM_PROD_ID 0x3 144 #define EEPROM_MFG_ID 0x7 145 #define EEPROM_ADDR_CFG 0x8 146 #define EEPROM_RESOURCE_CFG 0x9 157 #define EP_COMMAND 0x0e 159 #define EP_STATUS 0x0e 161 #define EP_WINDOW 0x0f 167 #define EP_W0_EEPROM_DATA 0x0c 168 #define EP_W0_EEPROM_COMMAND 0x0a 169 #define EP_W0_RESOURCE_CFG 0x08 170 #define EP_W0_ADDRESS_CFG 0x06 171 #define EP_W0_CONFIG_CTRL 0x04 173 #define EP_W0_PRODUCT_ID 0x02 174 #define EP_W0_MFG_ID 0x00 180 #define EP_W1_TX_PIO_WR_2 0x02 181 #define EP_W1_TX_PIO_WR_1 0x00 183 #define EP_W1_FREE_TX 0x0c 184 #define EP_W1_TX_STATUS 0x0b 185 #define EP_W1_TIMER 0x0a 186 #define EP_W1_RX_STATUS 0x08 187 #define EP_W1_RX_PIO_RD_2 0x02 188 #define EP_W1_RX_PIO_RD_1 0x00 194 #define EP_W2_ADDR_5 0x05 195 #define EP_W2_ADDR_4 0x04 196 #define EP_W2_ADDR_3 0x03 197 #define EP_W2_ADDR_2 0x02 198 #define EP_W2_ADDR_1 0x01 199 #define EP_W2_ADDR_0 0x00 205 #define EP_W3_FREE_TX 0x0c 206 #define EP_W3_FREE_RX 0x0a 212 #define EP_W4_MEDIA_TYPE 0x0a 213 #define EP_W4_CTRLR_STATUS 0x08 214 #define EP_W4_NET_DIAG 0x06 215 #define EP_W4_FIFO_DIAG 0x04 216 #define EP_W4_HOST_DIAG 0x02 217 #define EP_W4_TX_DIAG 0x00 223 #define EP_W5_READ_0_MASK 0x0c 224 #define EP_W5_INTR_MASK 0x0a 225 #define EP_W5_RX_FILTER 0x08 226 #define EP_W5_RX_EARLY_THRESH 0x06 227 #define EP_W5_TX_AVAIL_THRESH 0x02 228 #define EP_W5_TX_START_THRESH 0x00 234 #define TX_TOTAL_OK 0x0c 235 #define RX_TOTAL_OK 0x0a 236 #define TX_DEFERRALS 0x08 237 #define RX_FRAMES_OK 0x07 238 #define TX_FRAMES_OK 0x06 239 #define RX_OVERRUNS 0x05 240 #define TX_COLLISIONS 0x04 241 #define TX_AFTER_1_COLLISION 0x03 242 #define TX_AFTER_X_COLLISIONS 0x02 243 #define TX_NO_SQE 0x01 244 #define TX_CD_LOST 0x00 260 #define GLOBAL_RESET (u_short) 0x0000 262 #define WINDOW_SELECT (u_short) (0x1<<11) 263 #define START_TRANSCEIVER (u_short) (0x2<<11) 269 #define RX_DISABLE (u_short) (0x3<<11) 271 #define RX_ENABLE (u_short) (0x4<<11) 272 #define RX_RESET (u_short) (0x5<<11) 273 #define RX_DISCARD_TOP_PACK (u_short) (0x8<<11) 274 #define TX_ENABLE (u_short) (0x9<<11) 275 #define TX_DISABLE (u_short) (0xa<<11) 276 #define TX_RESET (u_short) (0xb<<11) 277 #define REQ_INTR (u_short) (0xc<<11) 278 #define SET_INTR_MASK (u_short) (0xe<<11) 279 #define SET_RD_0_MASK (u_short) (0xf<<11) 280 #define SET_RX_FILTER (u_short) (0x10<<11) 281 #define FIL_INDIVIDUAL (u_short) (0x1) 282 #define FIL_GROUP (u_short) (0x2) 283 #define FIL_BRDCST (u_short) (0x4) 284 #define FIL_ALL (u_short) (0x8) 285 #define SET_RX_EARLY_THRESH (u_short) (0x11<<11) 286 #define SET_TX_AVAIL_THRESH (u_short) (0x12<<11) 287 #define SET_TX_START_THRESH (u_short) (0x13<<11) 288 #define STATS_ENABLE (u_short) (0x15<<11) 289 #define STATS_DISABLE (u_short) (0x16<<11) 290 #define STOP_TRANSCEIVER (u_short) (0x17<<11) 295 #define ACK_INTR (u_short) (0x6800) 296 #define C_INTR_LATCH (u_short) (ACK_INTR|0x1) 297 #define C_CARD_FAILURE (u_short) (ACK_INTR|0x2) 298 #define C_TX_COMPLETE (u_short) (ACK_INTR|0x4) 299 #define C_TX_AVAIL (u_short) (ACK_INTR|0x8) 300 #define C_RX_COMPLETE (u_short) (ACK_INTR|0x10) 301 #define C_RX_EARLY (u_short) (ACK_INTR|0x20) 302 #define C_INT_RQD (u_short) (ACK_INTR|0x40) 303 #define C_UPD_STATS (u_short) (ACK_INTR|0x80) 304 #define C_MASK (u_short) 0xFF 324 #define S_INTR_LATCH (u_short) (0x1) 325 #define S_CARD_FAILURE (u_short) (0x2) 326 #define S_TX_COMPLETE (u_short) (0x4) 327 #define S_TX_AVAIL (u_short) (0x8) 328 #define S_RX_COMPLETE (u_short) (0x10) 329 #define S_RX_EARLY (u_short) (0x20) 330 #define S_INT_RQD (u_short) (0x40) 331 #define S_UPD_STATS (u_short) (0x80) 332 #define S_MASK (u_short) 0xFF 333 #define S_5_INTS (S_CARD_FAILURE|S_TX_COMPLETE|\ 334 S_TX_AVAIL|S_RX_COMPLETE|S_RX_EARLY) 335 #define S_COMMAND_IN_PROGRESS (u_short) (0x1000) 341 #define ACF_CONNECTOR_BITS 14 342 #define ACF_CONNECTOR_UTP 0 343 #define ACF_CONNECTOR_AUI 1 344 #define ACF_CONNECTOR_BNC 3 351 #define SET_IRQ(base,irq) outw((base) + EP_W0_RESOURCE_CFG, \ 352 ((inw((base) + EP_W0_RESOURCE_CFG) & 0x0fff) | \ 353 ((u_short)(irq)<<12)) ) 372 #define ERR_RX_INCOMPLETE (u_short) (0x1<<15) 373 #define ERR_RX (u_short) (0x1<<14) 374 #define ERR_RX_OVERRUN (u_short) (0x8<<11) 375 #define ERR_RX_RUN_PKT (u_short) (0xb<<11) 376 #define ERR_RX_ALIGN (u_short) (0xc<<11) 377 #define ERR_RX_CRC (u_short) (0xd<<11) 378 #define ERR_RX_OVERSIZE (u_short) (0x9<<11) 379 #define ERR_RX_DRIBBLE (u_short) (0x2<<11) 399 #define TXS_COMPLETE 0x80 400 #define TXS_SUCCES_INTR_REQ 0x40 401 #define TXS_JABBER 0x20 402 #define TXS_UNDERRUN 0x10 403 #define TXS_MAX_COLLISION 0x8 404 #define TXS_STATUS_OVERFLOW 0x4 411 #define IS_AUI (1<<13) 412 #define IS_BNC (1<<12) 413 #define IS_UTP (1<<9) 415 #define ENABLE_DRQ_IRQ 0x0001 416 #define W0_P4_CMD_RESET_ADAPTER 0x4 417 #define W0_P4_CMD_ENABLE_ADAPTER 0x1 422 #define ENABLE_UTP 0xc0 423 #define DISABLE_UTP 0x0 428 #define ACTIVATE_ADAPTER_TO_CONFIG 0xff 429 #define MFG_ID 0x6d50 430 #define PROD_ID 0x9150 436 #define RX_BYTES_MASK (u_short) (0x07ff)